js在body部分编写一个button按钮标签,为按钮添加点击事件,按钮点击后跳转到百度首页
时间: 2024-03-20 21:40:29 浏览: 24
好的,这里是您需要的代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Button Click Example</title>
</head>
<body>
<button onclick="window.location.href='https://www.baidu.com'">
点击跳转到百度首页
</button>
</body>
</html>
```
这段代码会创建一个按钮,当用户点击按钮时,会通过 JavaScript 代码将页面重定向到百度首页。
相关问题
js,test2body部分编写一个button按钮,按钮点击后跳转到test1
好的,以下是一个简单的例子,可以实现点击按钮跳转到test1页面:
```html
<!DOCTYPE html>
<html>
<head>
<title>跳转页面</title>
</head>
<body>
<button onclick="location.href='test1.html'">跳转到test1页面</button>
</body>
</html>
```
这段代码中,我们使用了HTML中的button元素,并为其添加了一个onclick事件。当用户点击按钮时,该事件会触发JavaScript代码,通过location.href属性将页面跳转到test1.html。
当然,你需要将该代码保存为一个HTML文件,并将test1.html文件放置在与该HTML文件同一目录下,才能正常访问test1页面。
用HTML写一个扫描之后有按钮的二维码点击按钮后跳转到CSDN的二维码
### 回答1:
你好!下面是一个使用 HTML 写成的二维码,扫描后会出现一个按钮,点击按钮后会跳转到 CSDN 的二维码:
```
<div id="qrcode">
<img src="https://api.qrserver.com/v1/create-qr-code/?size=150x150&data=https://www.csdn.net" alt="CSDN 二维码">
<button onclick="location.href='https://www.csdn.net'">跳转到 CSDN</button>
</div>
```
这个二维码图像是通过使用二维码生成 API 生成的,你也可以使用其他的二维码生成工具生成你自己的二维码图像。
希望这个答案对你有帮助!
### 回答2:
可以使用HTML编写一个带有按钮的二维码,点击按钮后可以跳转到CSDN的二维码链接。具体步骤如下:
1. 首先,我们需要生成CSDN的二维码图片。可以使用在线的二维码生成器,将CSDN的链接(例如https://www.csdn.net)生成为二维码图片。
2. 将生成的CSDN二维码图片保存到本地,记下图片的路径。
3. 使用HTML代码创建一个包含按钮和图片的页面。可以通过以下代码实现:
```html
<!DOCTYPE html>
<html>
<head>
<title>扫描二维码</title>
</head>
<body>
<button onclick="redirectToCSDN()">点击跳转到CSDN</button>
<br>
<img id="qrcode" src="二维码路径" alt="CSDN二维码">
<script>
function redirectToCSDN(){
window.location.href = "https://www.csdn.net"; // 点击按钮后跳转到CSDN链接
}
</script>
</body>
</html>
```
在上述代码中,将“二维码路径”替换为实际的CSDN二维码图片的路径。
4. 将上述代码保存为一个HTML文件,然后打开该文件,即可看到一个带有按钮和二维码的页面。
当用户扫描二维码后,点击按钮即可跳转到CSDN的链接页面。
### 回答3:
HTML是一种标记语言,用于构建网页和应用程序的前端界面。要实现在扫描二维码后显示一个按钮,并且点击按钮后跳转到CSDN的二维码,可以按照以下步骤进行:
1. 首先,需要生成一个包含CSDN链接的二维码。可以使用在线二维码生成器或者第三方库来创建二维码图片。
2. 使用HTML标记语言创建一个简单的网页。在网页中添加一个按钮和一个图片标签。
3. 给按钮和图片标签添加必要的属性和事件。
4. 在按钮的点击事件中,通过JavaScript代码实现跳转到CSDN链接的功能。
下面是一个示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>扫描二维码按钮跳转示例</title>
<script>
function goToCSDN() {
window.location.href = "https://www.csdn.net"; // 跳转至CSDN链接
}
</script>
</head>
<body>
<h1>扫描二维码后点击按钮跳转</h1>
<button onclick="goToCSDN()">跳转到CSDN</button> <!-- 点击按钮调用goToCSDN函数 -->
<br>
<img src="二维码图片的路径" alt="CSDN二维码"> <!-- 二维码图片的路径根据实际生成的二维码来确定 -->
</body>
</html>
```
在上述代码中,点击"跳转到CSDN"按钮时,将调用名为"goToCSDN"的JavaScript函数。该函数通过window.location.href将当前页面跳转至CSDN的链接。
需要注意的是,在代码中需要将"二维码图片的路径"替换为实际生成的二维码的图片路径。
相关推荐
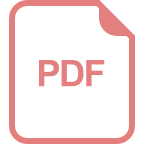
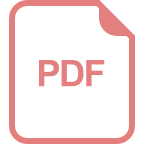












