使用c语言实现lru页面置换算法
时间: 2023-10-03 21:10:08 浏览: 101
好的,下面是使用C语言实现LRU页面置换算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 5 // 缓存最大大小
#define HIT 1 // 命中
#define MISS 0 // 未命中
// 双向链表节点
typedef struct _node {
int key; // 缓存的键
int value; // 缓存的值
struct _node *prev; // 前驱节点指针
struct _node *next; // 后继节点指针
} Node;
// 双向链表
typedef struct _list {
int count; // 缓存的节点数
Node *head; // 链表头指针
Node *tail; // 链表尾指针
} List;
// 缓存结构体
typedef struct _cache {
List *list; // 双向链表指针
int size; // 缓存大小
} Cache;
// 创建双向链表
List *createList() {
List *list = (List *)malloc(sizeof(List));
list->count = 0;
list->head = NULL;
list->tail = NULL;
return list;
}
// 创建节点
Node *createNode(int key, int value) {
Node *node = (Node *)malloc(sizeof(Node));
node->key = key;
node->value = value;
node->prev = NULL;
node->next = NULL;
return node;
}
// 添加节点到链表头部
void addToHead(List *list, Node *node) {
if (list->count == 0) {
list->head = node;
list->tail = node;
} else {
node->next = list->head;
list->head->prev = node;
list->head = node;
}
list->count++;
}
// 删除节点
void removeNode(List *list, Node *node) {
if (list->count == 0) {
return;
}
if (list->count == 1) {
list->head = NULL;
list->tail = NULL;
} else if (node == list->head) {
list->head = node->next;
list->head->prev = NULL;
} else if (node == list->tail) {
list->tail = node->prev;
list->tail->next = NULL;
} else {
node->prev->next = node->next;
node->next->prev = node->prev;
}
free(node);
list->count--;
}
// 删除链表尾部节点
void removeTail(List *list) {
if (list->count == 0) {
return;
}
Node *node = list->tail;
list->tail = node->prev;
if (list->count == 1) {
list->head = NULL;
list->tail = NULL;
} else {
list->tail->next = NULL;
}
free(node);
list->count--;
}
// 移动节点到链表头部
void moveToHead(List *list, Node *node) {
if (node == list->head) {
return;
}
if (node == list->tail) {
list->tail = node->prev;
list->tail->next = NULL;
} else {
node->prev->next = node->next;
node->next->prev = node->prev;
}
node->prev = NULL;
node->next = list->head;
list->head->prev = node;
list->head = node;
}
// 创建缓存
Cache *createCache(int size) {
Cache *cache = (Cache *)malloc(sizeof(Cache));
cache->list = createList();
cache->size = size;
return cache;
}
// 获取缓存值
int get(Cache *cache, int key, int *value) {
Node *node = cache->list->head;
while (node) {
if (node->key == key) {
*value = node->value;
moveToHead(cache->list, node);
return HIT;
}
node = node->next;
}
return MISS;
}
// 设置缓存值
void put(Cache *cache, int key, int value) {
Node *node = cache->list->head;
while (node) {
if (node->key == key) {
node->value = value;
moveToHead(cache->list, node);
return;
}
node = node->next;
}
node = createNode(key, value);
addToHead(cache->list, node);
if (cache->list->count > cache->size) {
removeTail(cache->list);
}
}
// 打印缓存
void printCache(Cache *cache) {
Node *node = cache->list->head;
while (node) {
printf("(%d, %d) ", node->key, node->value);
node = node->next;
}
printf("\n");
}
int main() {
Cache *cache = createCache(MAXSIZE);
int value;
put(cache, 1, 1);
printf("cache: ");
printCache(cache);
put(cache, 2, 2);
printf("cache: ");
printCache(cache);
put(cache, 3, 3);
printf("cache: ");
printCache(cache);
get(cache, 1, &value);
printf("get(1): %d\n", value);
printf("cache: ");
printCache(cache);
put(cache, 4, 4);
printf("cache: ");
printCache(cache);
put(cache, 5, 5);
printf("cache: ");
printCache(cache);
put(cache, 6, 6);
printf("cache: ");
printCache(cache);
get(cache, 3, &value);
printf("get(3): %d\n", value);
printf("cache: ");
printCache(cache);
return 0;
}
```
该示例代码实现了一个LRU缓存,支持添加、获取、打印缓存等操作。其中,缓存使用双向链表来实现,每当有新的元素被访问时,将其移动到链表头部,最近最少使用的元素将会被移动到链表尾部,并在缓存达到最大大小时被删除。
阅读全文
相关推荐
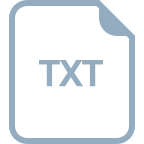
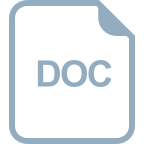



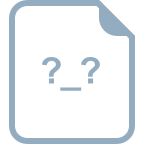
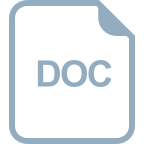
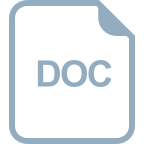
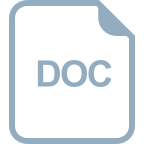
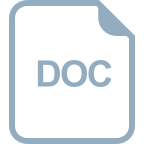
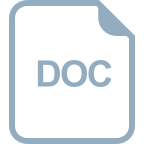
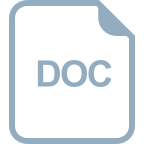




