def _resnet( block: Type[Union[BasicBlock, Bottleneck]], layers: List[int], weights: Optional[WeightsEnum], progress: bool, **kwargs: Any, ) -> ResNet: if weights is not None: _ovewrite_named_param(kwargs, "num_classes", len(weights.meta["categories"])) model = ResNet(block, layers, **kwargs) if weights is not None: model.load_state_dict(weights.get_state_dict(progress=progress, check_hash=True)) return model _COMMON_META = { "min_size": (1, 1), "categories": _IMAGENET_CATEGORIES, }
时间: 2024-04-26 15:25:16 浏览: 7
这段代码中的 `_resnet` 函数实现了一个 ResNet 网络的加载过程,其中 `ResNet` 类是一个 ResNet 网络的实现,`weights` 参数是用于加载预训练模型的权重,`progress` 参数用于控制是否显示下载进度条,`kwargs` 参数是一些其他的网络参数。函数会根据是否有预训练模型的权重来决定是否修改网络的输出类别数,然后返回加载好权重的模型。
此外,代码中还定义了一个 `_COMMON_META` 字典,用于存储一些常用的元数据信息,如图片的最小尺寸和类别信息。
相关问题
def _resnet(arch, block, layers, pretrained, progress, **kwargs):
这是一个函数定义,其中参数包括:
- arch:表示使用的 ResNet 模型结构,例如 ResNet-18、ResNet-50 等。
- block:表示 ResNet 中的基本块类型,通常是 BasicBlock 或 Bottleneck。
- layers:表示 ResNet 中每个阶段包含的基本块数量。
- pretrained:表示是否使用预训练的模型参数。
- progress:表示在下载模型参数时是否显示下载进度。
- **kwargs:表示可以传递其他参数给该函数。
该函数的作用是构建一个 ResNet 模型,并返回该模型的实例。
blocks_dict = { 'BASIC': BasicBlock, 'BOTTLENECK': Bottleneck }
这是一个字典,其键是字符串 'BASIC' 和 'BOTTLENECK',其值分别是 BasicBlock 和 Bottleneck 类的引用。这通常用于在深度学习模型中选择不同类型的基本块,比如 ResNet 中的基本块可以选择 BasicBlock 或 Bottleneck。通过使用这个字典,可以方便地在模型中指定使用哪种基本块。
相关推荐
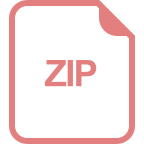
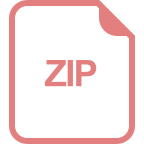












