编写程序,删除一个字符串中某个字符
时间: 2024-05-02 22:19:41 浏览: 97
您可以使用 Python 中的字符串 replace() 方法来删除字符串中的某个字符。例如:
```python
string = "hello world"
char_to_remove = "l"
new_string = string.replace(char_to_remove, "")
print(new_string)
```
输出将为:"heo word"
希望这个回答可以帮到您。
相关问题
用java编写编写程序求两个正整数的最大公约数。 9. 删除一个字符串中指定的所有某个字符,返回清理后的字符串。 n 输入字符串:aabbccddffeedd; n 删除字符:b,则程序输出:aaccddffeedd n 删除子串,只要是原串中有相同的子串就删掉,不管有多少个,返回子串个数和删除后的字符串
用Java编写程序求两个正整数的最大公约数:
```
public static int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
```
删除一个字符串中指定的所有某个字符,返回清理后的字符串:
```
public static String removeChar(String str, char c) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (ch != c) {
sb.append(ch);
}
}
return sb.toString();
}
```
输入字符串:aabbccddffeedd;删除字符:b,则程序输出:aaccddffeedd
```
String str = "aabbccddffeedd";
char c = 'b';
String result = removeChar(str, c);
System.out.println(result);
```
输出:aaccddffeedd
删除子串,只要是原串中有相同的子串就删掉,不管有多少个,返回子串个数和删除后的字符串:
```
public static int removeSubstr(String str, String substr) {
int count = 0;
int index;
while ((index = str.indexOf(substr)) != -1) {
count++;
str = str.substring(0, index) + str.substring(index + substr.length());
}
System.out.println("删除了" + count + "个子串,结果为:" + str);
return count;
}
```
输入字符串:aabbccddffeedd;删除子串:dd,则程序输出:删除了2个子串,结果为:aabbcceeffee
```
String str = "aabbccddffeedd";
String substr = "dd";
int count = removeSubstr(str, substr);
```
输出:删除了2个子串,结果为:aabbcceeffee
用java编写下列程序:从一个字符串中删除从某个特定字符开始的所有字符。例如原字符串为“abcdefg”,特定字符为“d”,删除后的字符串为“abc”。
下面是Java代码实现从一个字符串中删除从某个特定字符开始的所有字符:
```java
import java.util.Scanner;
public class DeleteChar {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("请输入原字符串:");
String str = input.nextLine();
System.out.print("请输入特定字符:");
char ch = input.next().charAt(0);
int index = str.indexOf(ch); // 获取特定字符的位置
if (index != -1) {
str = str.substring(0, index); // 删除特定字符及其后面的所有字符
}
System.out.println("删除后的字符串为:" + str);
}
}
```
运行程序,输入原字符串和特定字符,即可得到删除后的字符串。注意,如果特定字符不存在于原字符串中,输出结果不变。
阅读全文
相关推荐
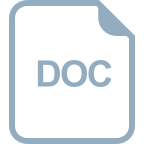
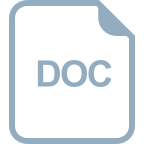
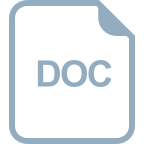












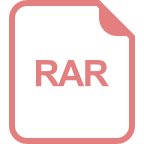

