用C语言写stm32 uart1接收uart2发送程序
时间: 2023-06-03 13:01:37 浏览: 261
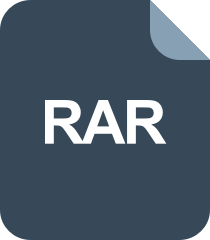
STM32F103ZET6核心板例程:UART测试程序源代码.rar
很高兴为你解决问题。以下是用C语言写stm32 uart1接收uart2发送程序的示例代码:
```
#include "stm32f10x.h"
#include <stdio.h>
void USART1_Init(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable USART1 and GPIOA clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
/* Configure PA9 and PA10 as USART1 Tx and Rx */
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* USART1 configuration */
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
/* Enable USART1 */
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART2_Init(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
/* Enable USART2 and GPIOA clock */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
/* Configure PA2 and PA3 as USART2 Tx and Rx */
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_3;
GPIO_Init(GPIOA, &GPIO_InitStructure);
/* USART2 configuration */
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
/* Enable USART2 */
USART_Init(USART2, &USART_InitStructure);
USART_Cmd(USART2, ENABLE);
}
void USART_SendChar(USART_TypeDef* USARTx, uint8_t ch)
{
/* Wait until transmit data register is empty */
while (USART_GetFlagStatus(USARTx, USART_FLAG_TXE) == RESET);
/* Send a character to USART */
USART_SendData(USARTx, (uint8_t) ch);
}
uint16_t USART_ReceiveData(USART_TypeDef* USARTx)
{
/* Wait until there is some data to receive */
while (USART_GetFlagStatus(USARTx, USART_FLAG_RXNE) == RESET);
/* Read received data from USART */
return (uint16_t) USART_ReceiveData(USARTx);
}
int main(void)
{
uint16_t data;
USART1_Init();
USART2_Init();
while (1)
{
/* Receive data from USART1 */
data = USART_ReceiveData(USART1);
/* Send received data to USART2 */
USART_SendChar(USART2, (uint8_t) data);
/* Delay to avoid overloading USART */
Delay(100);
}
}
```
注意:以上示例代码仅供参考。实际情况中,您需要根据自己的需要进行修改。
阅读全文
相关推荐
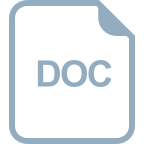
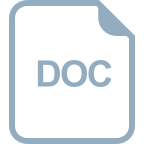
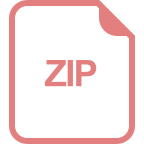
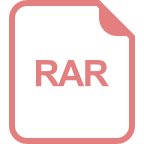
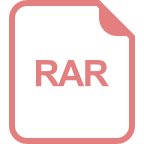








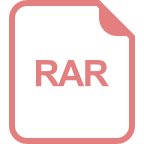
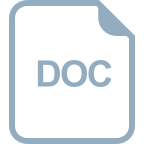
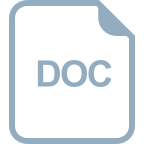

