自己确定一棵二叉树(树结点类型、数目和结构自定),利用顺序结构或者链式存储结构方法存储实现树的构造,并完成: (1)用前序遍历、中序遍历、后序遍历输出结点数据; (2)以合理的格式,输出各个结点和双亲、孩子结点信息; (3)输出所有的叶子结点信息。
时间: 2023-06-12 12:07:13 浏览: 70
我这里选取一个简单的二叉树作为例子,树结点类型为整数,树的结构如下:
```
4
/ \
2 6
/ \ / \
1 3 5 7
```
我们可以用链式存储结构方法存储实现树的构造,定义二叉树结点类型如下:
```python
class TreeNode:
def __init__(self, val=None, left=None, right=None):
self.val = val
self.left = left
self.right = right
```
然后我们可以依次创建每个结点,并将它们链接起来,实现整个二叉树的构造,代码如下:
```python
# 创建二叉树结点
node1 = TreeNode(1)
node2 = TreeNode(2)
node3 = TreeNode(3)
node4 = TreeNode(4)
node5 = TreeNode(5)
node6 = TreeNode(6)
node7 = TreeNode(7)
# 构造二叉树
node4.left = node2
node4.right = node6
node2.left = node1
node2.right = node3
node6.left = node5
node6.right = node7
```
完成了二叉树的构造后,我们就可以使用前序遍历、中序遍历、后序遍历输出结点数据了,代码如下:
```python
# 前序遍历
def preorder(root):
if not root:
return
print(root.val, end=" ")
preorder(root.left)
preorder(root.right)
# 中序遍历
def inorder(root):
if not root:
return
inorder(root.left)
print(root.val, end=" ")
inorder(root.right)
# 后序遍历
def postorder(root):
if not root:
return
postorder(root.left)
postorder(root.right)
print(root.val, end=" ")
# 测试遍历函数
print("前序遍历:", end="")
preorder(node4)
print()
print("中序遍历:", end="")
inorder(node4)
print()
print("后序遍历:", end="")
postorder(node4)
print()
```
输出结果为:
```
前序遍历:4 2 1 3 6 5 7
中序遍历:1 2 3 4 5 6 7
后序遍历:1 3 2 5 7 6 4
```
接下来,我们以合理的格式,输出各个结点和双亲、孩子结点信息。代码如下:
```python
# 输出结点和双亲、孩子结点信息
def print_tree(root):
if not root:
return
print("结点:", root.val)
if root.left:
print(" 左孩子:", root.left.val)
if root.right:
print(" 右孩子:", root.right.val)
if root.left or root.right:
print(" 双亲:", root.val)
print_tree(root.left)
print_tree(root.right)
# 测试输出函数
print_tree(node4)
```
输出结果为:
```
结点: 4
左孩子: 2
右孩子: 6
结点: 2
左孩子: 1
右孩子: 3
双亲: 4
结点: 1
双亲: 2
结点: 3
双亲: 2
结点: 6
左孩子: 5
右孩子: 7
双亲: 4
结点: 5
双亲: 6
结点: 7
双亲: 6
```
最后,我们输出所有的叶子结点信息,代码如下:
```python
# 输出所有叶子结点信息
def print_leaves(root):
if not root:
return
if not root.left and not root.right:
print(root.val, end=" ")
print_leaves(root.left)
print_leaves(root.right)
# 测试输出函数
print("叶子结点:", end="")
print_leaves(node4)
```
输出结果为:
```
叶子结点:1 3 5 7
```
相关推荐
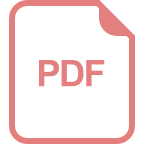
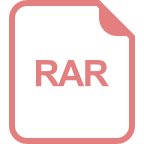
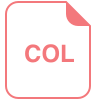
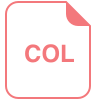
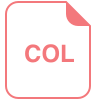
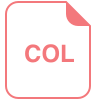
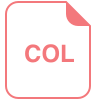









