使用动态内存分配创建封装的动态学生类Student的对象数组class,在主函数中计算出所有学生三门课程的平均成绩并输出
时间: 2023-12-03 10:42:03 浏览: 29
以下是使用动态内存分配创建封装的动态学生类Student的对象数组class,并计算所有学生三门课程的平均成绩的示例代码:
```cpp
#include <iostream>
using namespace std;
class Student {
private:
string name;
int score1, score2, score3;
public:
Student(string n, int s1, int s2, int s3): name(n), score1(s1), score2(s2), score3(s3) {}
double averageScore() const {
return (score1 + score2 + score3) / 3.0;
}
};
int main() {
int n;
cout << "Enter the number of students: ";
cin >> n;
Student* students = new Student[n];
for (int i = 0; i < n; i++) {
string name;
int score1, score2, score3;
cout << "Enter student " << i+1 << "'s name and three scores: ";
cin >> name >> score1 >> score2 >> score3;
students[i] = Student(name, score1, score2, score3);
}
double avg1 = 0, avg2 = 0, avg3 = 0;
for (int i = 0; i < n; i++) {
avg1 += students[i].averageScore() * students[i].score1;
avg2 += students[i].averageScore() * students[i].score2;
avg3 += students[i].averageScore() * students[i].score3;
}
avg1 /= n;
avg2 /= n;
avg3 /= n;
cout << "The average score for course 1 is " << avg1 << endl;
cout << "The average score for course 2 is " << avg2 << endl;
cout << "The average score for course 3 is " << avg3 << endl;
delete[] students;
return 0;
}
```
该示例代码首先从标准输入中读取学生数量,然后使用 `new` 运算符动态分配一个大小为 `n` 的 `Student` 数组,并循环读取每个学生的姓名和三门课程的成绩,将它们存储在相应的 `Student` 对象中。接着,循环遍历所有学生,计算每门课程的加权平均分,并输出结果。最后,使用 `delete` 运算符释放动态分配的内存。
需要注意的是,在实现 `averageScore()` 函数时,为了避免整数除法的问题,我们需要将求和结果强制转换为 `double` 类型,再除以 `3.0`。在计算加权平均分时,我们需要将每个学生的平均分乘以相应的课程分数,再累加到总分上,最后除以学生数量来求得加权平均分。
相关推荐
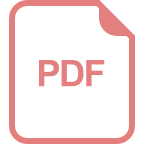














