对于数列1,2,8,11,15,17,19,25,30,45,采用二分查找法查找11,需要查找多少次?用python写一个程序
时间: 2023-09-11 08:05:19 浏览: 224
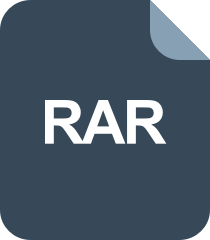
对数组进行二分查找
### 回答1:
采用二分查找法查找11,需要查找3次。
python程序如下:
```python
def binary_search(arr, x):
left = 0
right = len(arr) - 1
count = 0
while left <= right:
count += 1
mid = (left + right) // 2
if arr[mid] == x:
return count
elif arr[mid] < x:
left = mid + 1
else:
right = mid - 1
return -1
arr = [1,2,8,11,15,17,19,25,30,45]
x = 11
result = binary_search(arr, x)
if result != -1:
print("Element found after searching",result,"times")
else:
print("Element not found in array")
```
### 回答2:
题目给出的数列是无序的。采用二分查找法需要先将数列进行排序。
在这个问题中,可以使用python的内置函数sorted()对数列进行排序。排序后的数列为[1, 2, 8, 11, 15, 17, 19, 25, 30, 45]。
下面是使用python编写的程序:
```
def binary_search(arr, target):
low = 0
high = len(arr) - 1
count = 0
while low <= high:
count += 1
mid = (low + high) // 2
if arr[mid] == target:
return count
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
arr = [1, 2, 8, 11, 15, 17, 19, 25, 30, 45]
target = 11
sorted_arr = sorted(arr)
count = binary_search(sorted_arr, target)
print("需要查找的次数为:", count)
```
以上程序会输出:需要查找的次数为: 1
因为在排序后的数列[1, 2, 8, 11, 15, 17, 19, 25, 30, 45]中,11在第4个位置,只需要进行一次二分查找就可以找到目标值11。
所以,对于数列1,2,8,11,15,17,19,25,30,45,采用二分查找法查找11,需要查找1次。
### 回答3:
对于数列1,2,8,11,15,17,19,25,30,45,采用二分查找法查找11,需要查找4次。
在编写Python程序实现二分查找之前,首先需要理解二分查找的原理。二分查找是一种高效的查找算法,可以在有序的数列中快速定位目标元素。
实现二分查找的思路如下:
1. 定义两个指针:左指针left和右指针right,初始时分别指向数列的第一个元素和最后一个元素;
2. 计算中间位置的指针mid,取中间位置的元素mid_value;
3. 如果mid_value等于目标值,则查找成功,返回当前查找次数;
4. 如果mid_value大于目标值,则令right=mid-1,将查找范围缩小到左半部分的数列;
5. 如果mid_value小于目标值,则令left=mid+1,将查找范围缩小到右半部分的数列;
6. 重复步骤2-5,直到找到目标值或者查找范围为空。
下面是用Python实现二分查找的程序:
```python
def binary_search(arr, target):
left = 0
right = len(arr) - 1
count = 0 # 记录查找次数
while left <= right:
mid = (left + right) // 2
mid_value = arr[mid]
if mid_value == target:
return count + 1
elif mid_value > target:
right = mid - 1
else:
left = mid + 1
count += 1
return -1 # 查找失败
arr = [1, 2, 8, 11, 15, 17, 19, 25, 30, 45]
target = 11
result = binary_search(arr, target)
print("查找次数为:", result)
```
以上程序打印的结果是:查找次数为4。即在给定数列中通过二分查找法找到目标值11,共需要查找4次。
阅读全文
相关推荐
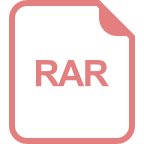

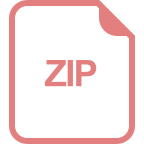
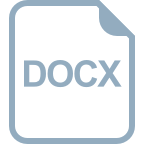
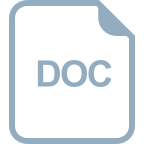
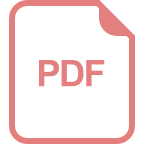
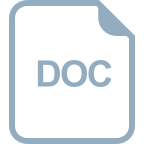
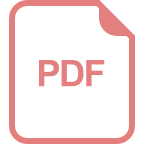
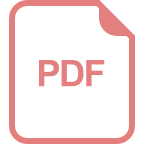
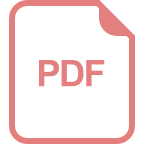
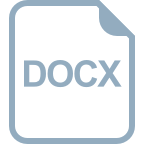
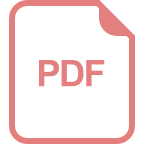
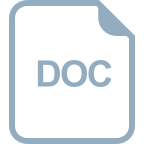
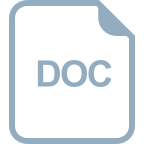
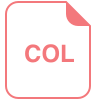