设计两个程序:有名管道的读程序和写程序,要求利用有名管道实现聊天程序,每次发言后自动在后面增加当前系统时间。增加结束字符,比如最后输入“886”后结束进程。
时间: 2024-05-14 18:19:28 浏览: 171
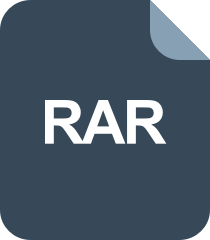
一个聊天程序
以下是有名管道的读程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <time.h>
#include <unistd.h>
#define FIFO_NAME "chat_fifo"
int main() {
int fd;
char buf[256];
// 创建有名管道
mkfifo(FIFO_NAME, 0666);
printf("等待连接...\n");
// 打开有名管道,以只读的方式读取数据
fd = open(FIFO_NAME, O_RDONLY);
printf("已连接!\n");
while (1) {
// 读取有名管道中的数据
if (read(fd, buf, sizeof(buf)) > 0) {
// 输出读取到的数据
printf("%s [读取自对方]\n", buf);
// 如果读取到的数据为结束符号,则退出
if (strcmp(buf, "886") == 0) {
break;
}
}
// 清空缓冲区
memset(buf, 0, sizeof(buf));
}
// 关闭有名管道
close(fd);
// 删除有名管道
unlink(FIFO_NAME);
printf("连接已断开!\n");
return 0;
}
```
以下是有名管道的写程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <time.h>
#include <unistd.h>
#define FIFO_NAME "chat_fifo"
int main() {
int fd;
char buf[256];
// 创建有名管道
mkfifo(FIFO_NAME, 0666);
printf("等待连接...\n");
// 打开有名管道,以只写的方式写入数据
fd = open(FIFO_NAME, O_WRONLY);
printf("已连接!\n");
while (1) {
// 读取用户输入的数据
fgets(buf, sizeof(buf), stdin);
// 在数据后面增加当前系统时间
time_t now = time(NULL);
struct tm *tm_now = localtime(&now);
char time_str[20];
strftime(time_str, sizeof(time_str), "[%H:%M:%S]", tm_now);
strcat(buf, time_str);
// 写入数据到有名管道中
write(fd, buf, strlen(buf) + 1);
// 如果写入的数据为结束符号,则退出
if (strcmp(buf, "886\n") == 0) {
break;
}
// 清空缓冲区
memset(buf, 0, sizeof(buf));
}
// 关闭有名管道
close(fd);
// 删除有名管道
unlink(FIFO_NAME);
printf("连接已断开!\n");
return 0;
}
```
编译并运行读程序和写程序,就可以实现通过有名管道进行聊天的功能了。
阅读全文
相关推荐
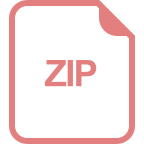
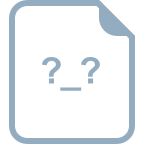



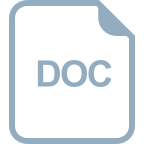
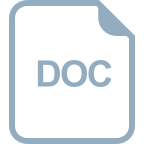
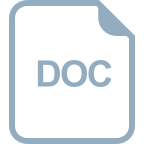
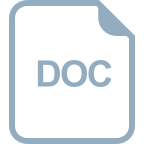
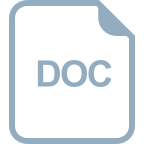




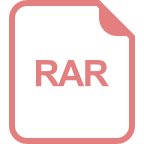
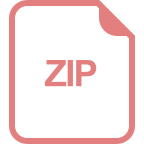
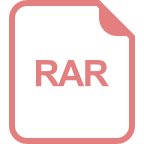