用C++中栈和队列的基本操作设计用两种以上的算法来实现判断输入的字符串序列是否为回文
时间: 2024-01-22 21:18:08 浏览: 35
算法一:使用栈
1. 将字符串依次压入栈中,即从字符串的第一个字符开始,依次将字符压入栈中,直到字符串结束。
2. 从字符串的第一个字符开始,依次将字符与栈顶元素进行比较,如果相等,则弹出栈顶元素,继续比较下一个字符;如果不相等,说明该字符串不是回文,直接返回false。
3. 如果比较完了所有字符,且栈已经为空,说明该字符串是回文,返回true;否则,说明该字符串不是回文,返回false。
代码如下:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool isPalindrome(string str) {
stack<char> s;
int len = str.length();
for (int i = 0; i < len; i++) {
s.push(str[i]);
}
for (int i = 0; i < len; i++) {
if (s.top() == str[i]) {
s.pop();
} else {
return false;
}
}
return true;
}
int main() {
string str;
cin >> str;
if (isPalindrome(str)) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
算法二:使用队列
1. 将字符串依次加入队列中,即从字符串的第一个字符开始,依次将字符加入队列中,直到字符串结束。
2. 从字符串的第一个字符开始,依次将字符与队列的队首元素进行比较,如果相等,则弹出队首元素,继续比较下一个字符;如果不相等,说明该字符串不是回文,直接返回false。
3. 如果比较完了所有字符,且队列已经为空,说明该字符串是回文,返回true;否则,说明该字符串不是回文,返回false。
代码如下:
```c++
#include <iostream>
#include <queue>
#include <string>
using namespace std;
bool isPalindrome(string str) {
queue<char> q;
int len = str.length();
for (int i = 0; i < len; i++) {
q.push(str[i]);
}
for (int i = 0; i < len; i++) {
if (q.front() == str[i]) {
q.pop();
} else {
return false;
}
}
return true;
}
int main() {
string str;
cin >> str;
if (isPalindrome(str)) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
以上两种算法都可以实现判断输入的字符串序列是否为回文,使用栈或队列都可以。但是,使用栈的空间复杂度更小,因为只需要一个栈就可以了,而使用队列则需要一个队列。
相关推荐
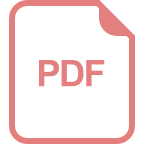
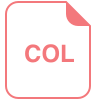
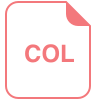
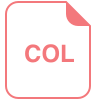
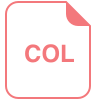
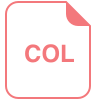









