springboot自定义redis注解AOP
时间: 2023-07-03 19:11:21 浏览: 70
好的,关于SpringBoot自定义Redis注解AOP的问题,我可以为您提供一些基本的介绍和示例代码。
Redis作为一种高性能的缓存和数据存储解决方案,被广泛应用于各种应用程序中。在SpringBoot应用程序中,使用Redis通常需要编写大量的重复代码,比如获取Redis连接、执行Redis命令、释放Redis连接等。这些重复代码不仅增加了开发和维护的难度,还影响了应用程序的性能。而AOP作为一种切面编程的技术,可以很好地解决这些问题。
下面是一个简单的示例代码,演示如何通过自定义注解实现对Redis操作的AOP处理:
首先,定义一个自定义注解:
```java
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface RedisCacheable {
String key() default "";
long expire() default 0;
}
```
然后,在需要被拦截的方法上添加该注解:
```java
@Component
public class RedisService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@RedisCacheable(key = "myKey", expire = 60)
public String getValue() {
return redisTemplate.opsForValue().get("myKey");
}
}
```
接下来,使用AspectJ的@Aspect注解定义一个切面类,并在该类中定义一个切点,用于匹配被@RedisCacheable注解的方法:
```java
@Aspect
@Component
public class RedisAspect {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@Pointcut("@annotation(com.example.demo.annotation.RedisCacheable)")
public void redisCacheablePointcut() {}
@Around("redisCacheablePointcut()")
public Object aroundRedisCacheable(ProceedingJoinPoint joinPoint) throws Throwable {
MethodSignature methodSignature = (MethodSignature) joinPoint.getSignature();
Method method = methodSignature.getMethod();
RedisCacheable redisCacheable = method.getAnnotation(RedisCacheable.class);
String key = redisCacheable.key();
long expire = redisCacheable.expire();
String value = redisTemplate.opsForValue().get(key);
if (value != null) {
return value;
}
Object result = joinPoint.proceed();
if (result != null) {
redisTemplate.opsForValue().set(key, result.toString());
if (expire > 0) {
redisTemplate.expire(key, expire, TimeUnit.SECONDS);
}
}
return result;
}
}
```
在该切面类中,使用@Around注解定义一个环绕通知,在该通知中,首先获取被拦截方法上的@RedisCacheable注解,然后根据注解中的key值从Redis中获取数据。如果Redis中已经存在该数据,则直接返回;否则,执行被拦截方法,并将结果存储到Redis缓存中。
最后,启动SpringBoot应用程序,调用RedisService的getValue方法,就可以看到输出结果:
```java
// 第一次调用,从数据库中获取数据,并将数据存入Redis缓存中
getValue...
// 第二次调用,直接从Redis中获取数据
getValue...
```
以上就是一个简单的SpringBoot自定义Redis注解AOP的示例。通过使用自定义注解和AOP技术,可以更加方便地实现对Redis缓存的操作,并提高应用程序的性能。
相关推荐
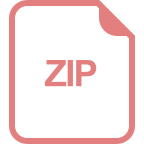
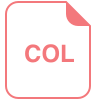












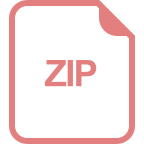
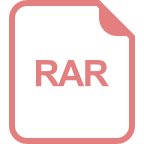