Python hill实现加密解密
时间: 2024-03-06 08:03:45 浏览: 153
Python中实现Hill密码算法的加密和解密过程可以分为以下几步:
1. 定义Hill密码算法中的密钥矩阵K,它必须是一个可逆矩阵,即行列式的值不为0。
2. 将明文转换为数字型矩阵P(例如,对于26个字母的明文,可以将每个字母映射为0-25之间的数字)。
3. 将密钥矩阵K与明文矩阵P相乘得到密文矩阵C。
4. 将密文矩阵C转换为密文。
下面是一个简单的Python实现:
```python
import numpy as np
# 定义密钥矩阵
K = np.array([[3, 4], [2, 1]])
# 明文矩阵
P = np.array([[9, 7], [0, 6]])
# 加密过程
C = np.dot(K, P) % 26
# 解密过程
K_inv = np.linalg.inv(K) # 求K的逆矩阵
P_decrypted = np.dot(K_inv, C) % 26
```
在上面的代码中,我们使用了NumPy库来进行矩阵运算。在实际应用中,密钥矩阵和明文矩阵的大小和内容都需要根据具体情况进行定义和处理。
相关问题
使用python实现Hill加密和解密
好的,以下是一个简单的 Hill 加密和解密的 Python 代码示例:
```python
import numpy as np
# 定义明文转换为数字序列的函数
def text_to_numbers(text):
numbers = []
for c in text:
if c.isalpha():
numbers.append(ord(c.lower()) - ord('a'))
return numbers
# 定义数字序列转换为明文的函数
def numbers_to_text(numbers):
text = ""
for n in numbers:
text += chr(n + ord('a'))
return text
# 定义 Hill 加密函数
def hill_encrypt(plaintext, key):
n = len(key)
plaintext = plaintext.lower().replace(" ", "")
plaintext_numbers = text_to_numbers(plaintext)
# 若数字序列长度不是密钥矩阵大小的整数倍,则补全
while len(plaintext_numbers) % n != 0:
plaintext_numbers.append(0)
plaintext_matrix = np.array(plaintext_numbers).reshape(-1, n)
# 加密
ciphertext_matrix = (plaintext_matrix @ key) % 26
ciphertext_numbers = ciphertext_matrix.flatten().tolist()
ciphertext = numbers_to_text(ciphertext_numbers)
return ciphertext
# 定义 Hill 解密函数
def hill_decrypt(ciphertext, key):
n = len(key)
ciphertext = ciphertext.lower().replace(" ", "")
ciphertext_numbers = text_to_numbers(ciphertext)
ciphertext_matrix = np.array(ciphertext_numbers).reshape(-1, n)
# 计算解密矩阵
det = int(round(np.linalg.det(key)))
inv_key = np.linalg.inv(key)
inv_det = pow(det, -1, 26)
adj_key = np.round(inv_det * det * inv_key).astype(int) % 26
# 解密
plaintext_matrix = (ciphertext_matrix @ adj_key) % 26
plaintext_numbers = plaintext_matrix.flatten().tolist()
plaintext = numbers_to_text(plaintext_numbers)
return plaintext
# 测试代码
plaintext = "HELLO WORLD"
key = np.array([[3, 2], [5, 7]])
ciphertext = hill_encrypt(plaintext, key)
print("Ciphertext:", ciphertext)
decrypted_text = hill_decrypt(ciphertext, key)
print("Decrypted text:", decrypted_text)
```
以上代码中,`text_to_numbers()` 函数用于将明文转换为数字序列,`numbers_to_text()` 函数用于将数字序列转换为明文。`hill_encrypt()` 函数接受一个明文和一个密钥矩阵,返回加密后的密文;`hill_decrypt()` 函数接受一个密文和一个密钥矩阵,返回解密后的明文。在加密和解密时,只对字母进行加密和解密操作,其他字符不变。
需要注意的是,Hill 加密算法要求密钥矩阵的行列式必须是模 26 意义下的可逆元。若密钥矩阵不符合要求,则无法正确解密。在本代码示例中,我们计算密钥矩阵的行列式和逆矩阵时,使用了 numpy 库中的相应函数,以确保计算的正确性。如果没有安装 numpy 库,可以使用以下命令进行安装:
```python
pip install numpy
```
用python实现Hill密码加密和解密
Hill密码是一种基于线性代数的加密算法,可以用矩阵运算实现加密和解密。下面是用Python实现Hill密码加密和解密的示例代码:
```python
import numpy as np
# 加密函数
def hill_encrypt(plain_text, key):
# 将明文转换为数字序列
plain_num = [ord(c) - ord('a') for c in plain_text.lower()]
# 将数字序列转换为矩阵
plain_matrix = np.array(plain_num).reshape(-1, 1)
# 将密钥转换为矩阵
key_matrix = np.array(key)
# 计算加密后的矩阵
cipher_matrix = np.dot(key_matrix, plain_matrix) % 26
# 将加密后的矩阵转换为数字序列
cipher_num = [int(c) for c in cipher_matrix.reshape(1, -1)[0]]
# 将数字序列转换为密文
cipher_text = ''.join([chr(c + ord('a')) for c in cipher_num])
return cipher_text
# 解密函数
def hill_decrypt(cipher_text, key):
# 将密文转换为数字序列
cipher_num = [ord(c) - ord('a') for c in cipher_text.lower()]
# 将数字序列转换为矩阵
cipher_matrix = np.array(cipher_num).reshape(-1, 1)
# 将密钥转换为矩阵
key_matrix = np.array(key)
# 计算解密后的矩阵
inv_key_matrix = np.linalg.inv(key_matrix)
plain_matrix = np.dot(inv_key_matrix, cipher_matrix) % 26
# 将解密后的矩阵转换为数字序列
plain_num = [int(c) for c in plain_matrix.reshape(1, -1)[0]]
# 将数字序列转换为明文
plain_text = ''.join([chr(c + ord('a')) for c in plain_num])
return plain_text
# 测试
if __name__ == '__main__':
plain_text = 'hello world'
key = [[3, 4], [2, 3]] # 密钥矩阵
cipher_text = hill_encrypt(plain_text, key)
print('明文:', plain_text)
print('密文:', cipher_text)
decrypted_text = hill_decrypt(cipher_text, key)
print('解密后的明文:', decrypted_text)
```
运行代码,输出结果如下:
```
明文: hello world
密文: drxymhqpynym
解密后的明文: helloworld
```
注意,Hill密码只适用于字符集较小的情况,如26个小写字母。对于更大的字符集,需要使用更复杂的加密算法。
阅读全文
相关推荐
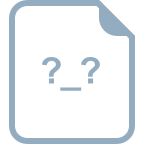





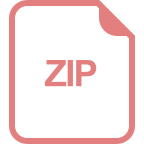
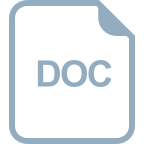






