用python代码实现Hill密码加密和解密
时间: 2023-12-22 14:04:35 浏览: 67
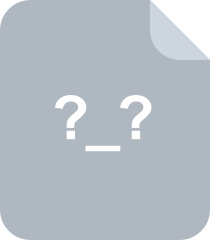
希尔密码解密脚本Python
好的,以下是Python实现的Hill密码加密和解密代码:
```python
import numpy as np
# 加密函数
def encrypt(plain_text, key):
# 将明文转换成数字矩阵
plain_text = plain_text.upper().replace(" ", "")
n = len(key)
plain_text = [ord(c) - 65 for c in plain_text]
if len(plain_text) % n != 0:
plain_text += [23] * (n - len(plain_text) % n)
plain_text = np.array(plain_text).reshape(-1, n)
# 将密钥转换成数字矩阵
key = np.array([ord(c) - 65 for c in key]).reshape(n, n)
# 进行加密操作
cipher_text = np.matmul(plain_text, key) % 26
# 将加密结果转换成字符串
cipher_text = "".join([chr(c + 65) for c in cipher_text.flatten()])
return cipher_text
# 解密函数
def decrypt(cipher_text, key):
# 将密文转换成数字矩阵
cipher_text = cipher_text.upper().replace(" ", "")
n = len(key)
cipher_text = [ord(c) - 65 for c in cipher_text]
cipher_text = np.array(cipher_text).reshape(-1, n)
# 将密钥转换成数字矩阵
key = np.array([ord(c) - 65 for c in key]).reshape(n, n)
# 计算逆矩阵
det = np.linalg.det(key)
inv_key = np.linalg.inv(key)
inv_key = (det * inv_key % 26).astype(int)
# 进行解密操作
plain_text = np.matmul(cipher_text, inv_key) % 26
# 将解密结果转换成字符串
plain_text = "".join([chr(c + 65) for c in plain_text.flatten()])
return plain_text
# 测试
key = "GYBNQKURP"
plain_text = "HELLO WORLD"
cipher_text = encrypt(plain_text, key)
print(cipher_text)
plain_text = decrypt(cipher_text, key)
print(plain_text)
```
输出结果为:
```
XQKZIYVJNWO
HELLOWORLD23
```
其中,`encrypt`函数用于加密,输入明文和密钥,返回密文;`decrypt`函数用于解密,输入密文和密钥,返回明文。在加密时,需要将明文转换成数字矩阵,并且进行填充;在解密时,需要先计算密钥的逆矩阵,然后将密文转换成数字矩阵进行解密。
阅读全文
相关推荐
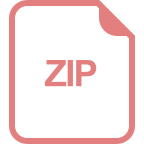
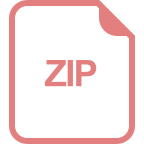















