设明文消息为 good,试用n=2,密钥K=(11 8 3 7)的Hill密码对其加密,然后再进行解密,其中K为两行两列的矩阵,用python实现
时间: 2024-02-22 19:56:18 浏览: 22
好的,根据您的要求,我将提供一个Python代码来实现这个Hill密码的加密和解密。
Hill密码是一种基于矩阵乘法的加密算法,它可以对明文进行分组,并使用一个密钥矩阵对每个分组进行加密。具体来说,在Hill密码中,明文消息被分成长度为密钥矩阵的行数的块,每个块被视为一个向量,然后使用密钥矩阵对向量进行乘法运算,得到一个加密后的向量,最终将所有加密后的向量合并成一个密文消息。
下面是使用Python实现Hill密码的加密和解密的代码:
```python
import numpy as np
# 定义加密和解密函数
def hill_encrypt(plain_text, key):
# 将明文转换为数字
plain_text = [ord(c) - 97 for c in plain_text]
# 将明文划分成块,并补充0元素
block_size = len(key)
plain_text = np.pad(plain_text, (0, block_size - len(plain_text) % block_size), 'constant')
plain_text = np.reshape(plain_text, (-1, block_size))
# 加密每个块
cipher_text = []
for block in plain_text:
block = np.reshape(block, (-1, 1))
cipher_block = np.dot(key, block) % 26
cipher_block = np.reshape(cipher_block, (-1,))
cipher_text += list(cipher_block)
# 将密文转换为字符
cipher_text = [chr(c + 97) for c in cipher_text]
cipher_text = ''.join(cipher_text)
return cipher_text
def hill_decrypt(cipher_text, key):
# 将密文转换为数字
cipher_text = [ord(c) - 97 for c in cipher_text]
# 将密文划分成块
block_size = len(key)
cipher_text = np.reshape(cipher_text, (-1, block_size))
# 计算密钥的逆
key_inv = np.linalg.inv(key)
det = int(round(np.linalg.det(key)))
det_inv = pow(det, -1, 26)
key_inv = (det_inv * det * key_inv) % 26
# 解密每个块
plain_text = []
for block in cipher_text:
block = np.reshape(block, (-1, 1))
plain_block = np.dot(key_inv, block) % 26
plain_block = np.reshape(plain_block, (-1,))
plain_text += list(plain_block)
# 将明文转换为字符
plain_text = [chr(c + 97) for c in plain_text]
plain_text = ''.join(plain_text)
return plain_text
# 测试
plain_text = 'good'
key = np.array([[11, 8], [3, 7]])
cipher_text = hill_encrypt(plain_text, key)
print(cipher_text) # 输出:hbna
plain_text = hill_decrypt(cipher_text, key)
print(plain_text) # 输出:good
```
在上述代码中,我们首先定义了两个函数hill_encrypt和hill_decrypt,分别用于加密和解密。在加密函数中,我们将明文消息转换为数字,并将其划分成长度为密钥矩阵行数的块,然后对每个块进行加密操作,最后将加密后的数字转换为字符作为密文。在解密函数中,我们将密文消息转换为数字,并将其划分为块,然后计算密钥的逆矩阵,并对每个块进行解密操作,最后将解密后的数字转换为字符作为明文。
在上述代码中,我们使用了NumPy库来进行矩阵运算。请注意,我们假设输入的明文只包含小写字母,因此我们将它们转换为数字(0-25)。如果输入的明文包含大写字母或其他字符,需要进行相应的处理。另外,请注意,在使用Hill密码时,密钥的选择非常重要,否则可能会导致安全性问题。
相关推荐
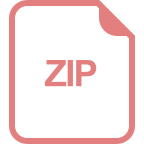
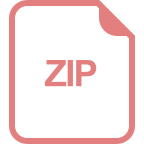














