成员函数实现Stack类: Push() -将元素添加到堆栈的顶部。pop) -从堆栈中删除顶部元素 Top() -返回堆栈中顶部的元素。
时间: 2024-01-21 20:18:30 浏览: 26
以下是一个基本的Stack类的实现,包括Push()、Pop()和Top()函数:
```c++
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 堆栈最大容量
class Stack {
private:
int data[MAX_SIZE]; // 存储堆栈元素的数组
int top; // 堆栈顶部元素在数组中的下标
public:
Stack() { // 构造函数
top = -1;
}
void Push(int x) { // 添加元素到堆栈顶部
if (top == MAX_SIZE - 1) { // 堆栈已满,不能继续添加元素
cout << "Error: Stack is full." << endl;
return;
}
data[++top] = x; // 将元素添加到堆栈的顶部
}
void Pop() { // 从堆栈中删除顶部元素
if (top == -1) { // 堆栈为空,不能执行删除操作
cout << "Error: Stack is empty." << endl;
return;
}
top--; // 删除堆栈顶部元素
}
int Top() { // 返回堆栈中顶部的元素
if (top == -1) { // 堆栈为空,不能返回元素
cout << "Error: Stack is empty." << endl;
return -1;
}
return data[top]; // 返回堆栈顶部元素
}
};
int main() {
Stack s;
s.Push(1);
s.Push(2);
s.Push(3);
cout << s.Top() << endl; // 输出3
s.Pop();
cout << s.Top() << endl; // 输出2
s.Pop();
cout << s.Top() << endl; // 输出1
s.Pop();
s.Pop(); // 尝试从空堆栈中删除元素,输出"Error: Stack is empty."
return 0;
}
```
在这个实现中,我们使用一个大小为MAX_SIZE的数组data来存储堆栈元素。top变量指示堆栈顶部元素在数组中的下标,初始化为-1表示堆栈为空。Push()函数将元素添加到堆栈顶部,Pop()函数从堆栈中删除顶部元素,Top()函数返回堆栈中顶部的元素。如果堆栈已满或者为空,这些函数会输出错误信息。在main()函数中,我们创建了一个Stack对象s,并使用Push()、Pop()和Top()函数测试它们的功能。
相关推荐
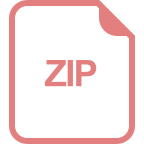
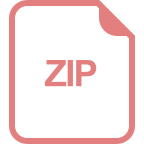
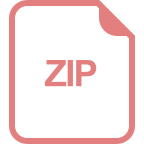














