C++重载运算符
时间: 2023-06-29 16:10:02 浏览: 115
C++中的运算符重载是一种特殊的函数重载,允许我们重新定义已有的运算符,使其能够用于自定义类型。运算符重载函数的名称必须是 `operator` 关键字后跟要重载的运算符的符号。
以下是一些常用的运算符及其重载函数的示例:
### 1. 算术运算符
| 运算符 | 函数名称 |
|-------|---------|
| + | operator+ |
| - | operator- |
| * | operator* |
| / | operator/ |
| % | operator% |
```cpp
class Number {
public:
int value;
Number(int v) : value(v) {}
Number operator+(const Number& other) {
return Number(value + other.value);
}
Number operator-(const Number& other) {
return Number(value - other.value);
}
Number operator*(const Number& other) {
return Number(value * other.value);
}
Number operator/(const Number& other) {
return Number(value / other.value);
}
Number operator%(const Number& other) {
return Number(value % other.value);
}
};
```
### 2. 比较运算符
| 运算符 | 函数名称 |
|-------|---------|
| == | operator== |
| != | operator!= |
| < | operator< |
| > | operator> |
| <= | operator<= |
| >= | operator>= |
```cpp
class Person {
public:
std::string name;
int age;
Person(const std::string& n, int a) : name(n), age(a) {}
bool operator==(const Person& other) {
return name == other.name && age == other.age;
}
bool operator!=(const Person& other) {
return !(*this == other);
}
bool operator<(const Person& other) {
return age < other.age;
}
bool operator>(const Person& other) {
return age > other.age;
}
bool operator<=(const Person& other) {
return age <= other.age;
}
bool operator>=(const Person& other) {
return age >= other.age;
}
};
```
### 3. 位运算符
| 运算符 | 函数名称 |
|-------|---------|
| & | operator& |
| \| | operator\| |
| ^ | operator^ |
| ~ | operator~ |
| << | operator<< |
| >> | operator>> |
```cpp
class Bitset {
private:
int data;
public:
Bitset(int d) : data(d) {}
Bitset operator&(const Bitset& other) {
return Bitset(data & other.data);
}
Bitset operator|(const Bitset& other) {
return Bitset(data | other.data);
}
Bitset operator^(const Bitset& other) {
return Bitset(data ^ other.data);
}
Bitset operator~() {
return Bitset(~data);
}
Bitset operator<<(int shift) {
return Bitset(data << shift);
}
Bitset operator>>(int shift) {
return Bitset(data >> shift);
}
};
```
阅读全文
相关推荐









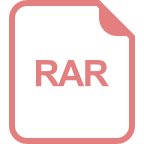




