编程有5个学生,每个学生有3门课的成绩,从键盘输入以上数据(包括学号,姓名,三门课成绩),计算出总成绩,将原有的数据和计算出的总分数存放在磁盘文件stu.txt中。 提示:首先以写方式打开文件stu.txt,由于学生人数已知,所以用for循环,对每个学生的信息从键盘输入,然后用fprintf()函数写入文件,最后关闭文件。
时间: 2024-01-03 11:03:17 浏览: 65
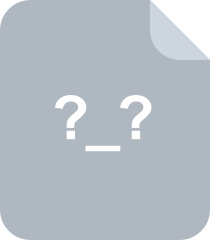
C语言程序设计-输入2个学生3门课的成绩,计算学生成绩平均分,课程平均分和最高分.

好的,这是一个关于文件操作和字符串处理的简单编程题目。下面是 C 语言的代码实现:
```c
#include <stdio.h>
#include <string.h>
#define NUM_STUDENTS 5
#define NUM_COURSES 3
struct Student {
char id[10];
char name[20];
int scores[NUM_COURSES];
int total_score;
};
int main() {
FILE *fp;
struct Student students[NUM_STUDENTS];
fp = fopen("stu.txt", "w");
if (fp == NULL) {
printf("File open error!\n");
return 1;
}
// 从键盘输入学生信息
for (int i=0; i<NUM_STUDENTS; i++) {
printf("Please input Student %d's ID: ", i+1);
scanf("%s", students[i].id);
printf("Please input Student %d's name: ", i+1);
scanf("%s", students[i].name);
printf("Please input Student %d's scores for 3 courses: ", i+1);
for (int j=0; j<NUM_COURSES; j++) {
scanf("%d", &students[i].scores[j]);
students[i].total_score += students[i].scores[j];
}
// 将学生信息写入文件
fprintf(fp, "%s %s", students[i].id, students[i].name);
for (int j=0; j<NUM_COURSES; j++) {
fprintf(fp, " %d", students[i].scores[j]);
}
fprintf(fp, " %d\n", students[i].total_score);
}
fclose(fp);
return 0;
}
```
这个程序可以从键盘输入学生信息,计算每个学生的总成绩,并将所有信息写入磁盘文件 `stu.txt` 中。在程序运行结束后,可以通过查看该文件的内容来验证信息是否正确存储。
阅读全文
相关推荐
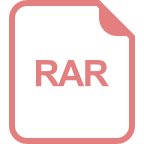
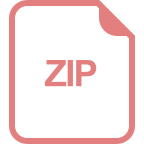













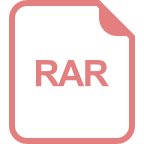
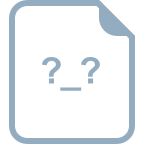
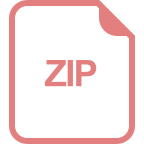