用matlab设计一个递归函数文件pow(x,m):xm,并在命令窗口中分别输出表达式2的5次方、(-3)的4次方、5的-2次方的值。
时间: 2024-02-21 21:02:17 浏览: 30
好的,以下是MATLAB的代码实现:
```matlab
function y = pow(x, m)
if m == 0
y = 1;
elseif m > 0
y = x * pow(x, m-1);
else
y = pow(x, m+1) / x;
end
end
```
这个函数的实现原理是通过递归实现幂运算。如果指数m为0,则结果为1;如果指数m为正数,则将运算分解为x乘以x的m-1次幂;如果指数m为负数,则将运算分解为x的-m次幂等于1除以x的m次幂。
接下来,你可以在命令窗口中分别输出表达式2的5次方、(-3)的4次方、5的-2次方的值。以下是示例代码:
```matlab
x = 2;
m = 5;
y = pow(x, m);
fprintf("%d的%d次方为:%d\n", x, m, y);
x = -3;
m = 4;
y = pow(x, m);
fprintf("(%d)的%d次方为:%d\n", x, m, y);
x = 5;
m = -2;
y = pow(x, m);
fprintf("%d的%d次方为:%f\n", x, m, y);
```
你只需要将以上三段代码放在同一个脚本文件中,运行即可得到结果。
相关问题
用matlab设计一个递归函数文件gcdw(ab):求整数a与b的最大公约数,并在脚本文件中输入整数a与整数b的值,并输出它们的最大公约数。
好的,以下是MATLAB的代码实现:
```matlab
function g = gcdw(a, b)
if b == 0
g = a;
else
g = gcdw(b, mod(a, b));
end
end
```
这个函数的实现原理与Python的代码实现是一样的,也是通过辗转相除法求最大公约数。
接下来,你可以在脚本文件中输入整数a与整数b的值,并输出它们的最大公约数。以下是示例代码:
```matlab
a = input("请输入整数a: ");
b = input("请输入整数b: ");
g = gcdw(a, b);
fprintf("整数a与整数b的最大公约数为:%d\n", g);
```
你只需要将以上两段代码放在同一个脚本文件中,运行即可得到结果。
C语言:编写递归函数求x的n次方, 并调用此函数求2的5次方。
好的,这是一个关于 C 语言的编程问题。可以使用递归算法来实现求 x 的 n 次方的功能。以下是一个示例代码:
```
#include <stdio.h>
double power(double x, int n)
{
if (n == 0)
{
return 1.0;
}
else if (n == 1)
{
return x;
}
else
{
double t = power(x, n/2);
if (n%2 == 0)
{
return t*t;
}
else
{
return t*t*x;
}
}
}
int main()
{
double result = power(2.0, 5);
printf("2^5 = %f\n", result);
return 0;
}
```
输出结果为:
```
2^5 = 32.000000
```
相关推荐
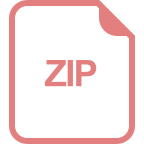
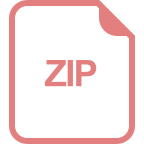
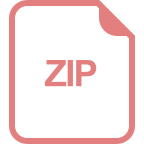












