public class IdiomProcessor { public static void main(String[] args) { // 读取文件 File file = new File("D:\\yy\\java\\成语接龙\\成语大全\\去重后.txt"); BufferedReader reader = null; HashMap<String, String> idiomMap = new HashMap<String, String>(); try { reader = new BufferedReader(new FileReader(file)); String line; while ((line = reader.readLine()) != null) { String[] parts = line.split("拼音:"); if(parts.length==2) { String idiom = parts[0].trim(); String pinyin = parts[1]; idiomMap.put(idiom, pinyin); } } } catch (IOException e) { e.printStackTrace(); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { e.printStackTrace(); } } // 统计末尾字出现次数 HashMap<String, Integer> countMap = new HashMap<String, Integer>(); for (String idiom : idiomMap.keySet()) { int count=0; for(String otheridiom:idiomMap.keySet()) if(otheridiom.startsWith(idiom.substring(idiom.length() - 1))) count++; countMap.put(idiom, count); //System.out.println(idiom+":"+count); } // 输出结果到文件 File outFile = new File("D:\\yy\\java\\成语接龙\\成语大全\\全量成语处理后.txt"); BufferedWriter writer = null; try { writer = new BufferedWriter(new FileWriter(outFile)); for (String idiom : idiomMap.keySet()) { String pinyin = idiomMap.get(idiom); writer.write(idiom + "拼音:" + pinyin + "可接:" + countMap.get(idiom) + "\n"); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (writer != null) { writer.close(); } } catch (IOException e) { e.printStackTrace(); } } } }解读代码
时间: 2024-02-10 18:22:47 浏览: 53
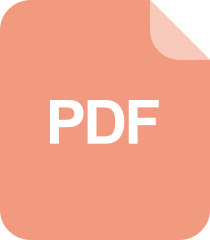
java中File类的构造函数及其方法
这段 Java 代码实现了一个成语接龙处理器。它的主要功能是读取一个成语库文件,统计每个成语末尾字在整个成语库中出现的次数,并将结果输出到文件中。下面是代码的详细解读:
1. 首先定义了一个名为 IdiomProcessor 的类,并在其中定义了一个名为 main 的静态方法。
2. 在 main 方法中,代码首先读取一个成语库文件,这个文件的路径为 "D:\\yy\\java\\成语接龙\\成语大全\\去重后.txt"。同时,定义了一个 HashMap 对象 idiomMap,用来存储每个成语及其拼音信息。
3. 在读取文件的过程中,代码按行读取文件内容,并使用 split 方法将每行内容按照 "拼音:" 进行分割,分割后的第一个部分为成语,第二个部分为成语的拼音。这些成语和拼音信息被存储到 idiomMap 中。
4. 读取文件完成后,代码开始统计每个成语的末尾字在整个成语库中出现的次数,并将统计结果存储到一个新的 HashMap 对象 countMap 中。具体实现是使用两层循环,对于每个成语,遍历整个成语库,统计以当前成语末尾字开头的成语数量。
5. 统计完成后,代码将结果输出到一个新的文件中,文件路径为 "D:\\yy\\java\\成语接龙\\成语大全\\全量成语处理后.txt"。同时,使用 BufferedWriter 对象 writer 进行文件写入操作。遍历 idiomMap 中每个成语,将成语、拼音和统计结果以指定格式写入文件中。
6. 最后,关闭 reader 和 writer 对象,完成整个处理过程。
阅读全文
相关推荐
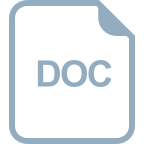
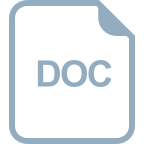
















