已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:在链表的倒数第k个位置插入元素x(即x成为链表的倒数第k个元素),插入成功返回1,不成功返回0(假设链表结点都在堆区)。函数原型为:int fun(Node *h, int x, int k)
时间: 2024-03-25 22:38:45 浏览: 54
好的,我来帮你解决这个问题。
首先,我们需要遍历整个链表,找到链表的长度len。因为要在倒数第k个位置插入元素,所以需要知道倒数第k个节点的前一个节点,即第len-k个节点。如果k大于等于链表长度,那么就无法插入,返回0即可。
接着,我们从链表头开始遍历,找到第len-k个节点p和它的前一个节点q。然后我们新建一个节点s,其data为x,next为p。最后,将q的next指向s,插入完成。
下面是完整的代码实现:
```c
int fun(Node *h, int x, int k) {
int len = 0;
Node *p = h, *q = h;
while (p->next) {
len++;
p = p->next;
if (len > k) {
q = q->next;
}
}
if (k >= len) {
return 0;
}
Node *s = (Node*)malloc(sizeof(Node));
s->data = x;
s->next = q->next;
q->next = s;
return 1;
}
```
注意在最后插入完成后,需要返回1表示插入成功。同时,由于涉及到动态内存分配,插入完成后还需要手动释放插入的节点。
相关问题
1. 已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:合并两个有序链表,使之仍然有序。函数原型为:Node * merge(Node*h1,Node*h2); 2. 2. 已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:在链表的倒数第k个位置插入元素x(即x成为链表的倒数第k个元素),插入成功返回链表头指针(链表第一个结点的地址),不成功返回NULL(假设链表结点都在堆区)。函数原型为:Node * fun(Node *h, int x, int k); 3. 设链表结点结构已经给出,试编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程。 typedef struct node { char Name[20]; struct node *Next; }Node;
1. 合并两个有序链表的函数实现如下:
```
Node* merge(Node* h1, Node* h2) {
if (!h1) {
return h2;
}
if (!h2) {
return h1;
}
if (h1->data < h2->data) {
h1->next = merge(h1->next, h2);
return h1;
} else {
h2->next = merge(h1, h2->next);
return h2;
}
}
```
2. 在链表的倒数第k个位置插入元素x的函数实现如下:
```
Node* fun(Node* h, int x, int k) {
Node* p = h;
Node* q = h;
for (int i = 0; i < k; i++) {
if (!q) {
return NULL;
}
q = q->next;
}
while (q) {
p = p->next;
q = q->next;
}
Node* node = (Node*) malloc(sizeof(Node));
node->data = x;
node->next = p->next;
p->next = node;
return h;
}
```
3. 插入、打印和销毁链表的函数实现如下:
```
void Insert(Node** head, char name[]) {
Node* node = (Node*) malloc(sizeof(Node));
strcpy(node->Name, name);
node->Next = NULL;
if (*head == NULL) {
*head = node;
} else {
Node* p = *head;
while (p->Next) {
p = p->Next;
}
p->Next = node;
}
}
void Print(Node* head) {
Node* p = head;
while (p) {
printf("%s ", p->Name);
p = p->Next;
}
printf("\n");
}
void Destroy(Node** head) {
Node* p = *head;
while (p) {
Node* q = p->Next;
free(p);
p = q;
}
*head = NULL;
}
```
完整调用过程如下:
```
int main() {
Node* head = NULL;
Insert(&head, "Alice");
Insert(&head, "Bob");
Insert(&head, "Charlie");
Print(head);
Destroy(&head);
return 0;
}
```
已知链表结构为:typedef struct Node{int data; struct Node *next;}Node;编写函数实现:合并两个有序链表,使之仍然有序。函数原型为:Node * merge(Node*h1,Node*h2);
下面是实现合并两个有序链表的代码:
```
Node * merge(Node*h1,Node*h2) {
Node *head = NULL;
Node *tail = NULL;
while (h1 != NULL && h2 != NULL) {
if (h1->data <= h2->data) {
if (head == NULL) {
head = tail = h1;
} else {
tail->next = h1;
tail = h1;
}
h1 = h1->next;
} else {
if (head == NULL) {
head = tail = h2;
} else {
tail->next = h2;
tail = h2;
}
h2 = h2->next;
}
}
if (h1 != NULL) {
if (head == NULL) {
head = h1;
} else {
tail->next = h1;
}
}
if (h2 != NULL) {
if (head == NULL) {
head = h2;
} else {
tail->next = h2;
}
}
return head;
}
```
该函数接收两个有序链表的头指针 `h1` 和 `h2`,返回一个新的有序链表的头指针。该函数使用双指针法,从头开始遍历两个有序链表,比较当前节点的值大小,将小的节点插入新链表中,直到其中一个链表遍历完毕。最后将剩余的链表直接插入到新链表的末尾即可。
阅读全文
相关推荐
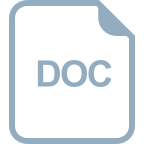
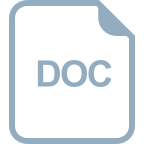
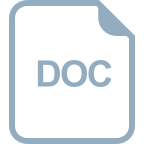










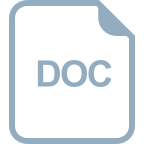
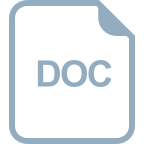
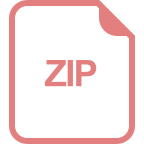
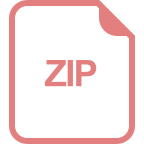
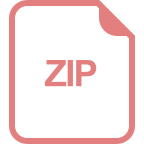