c++语言实现单链表的交并
时间: 2023-05-17 16:07:52 浏览: 82
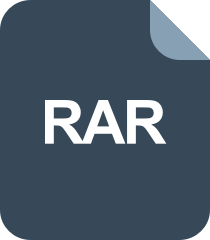
数据结构与算法 c++实现 两个单链表合并为一个单链表,两个表的元素交错排列,新表用原表结点的内存空间 按元素序列的奇偶分割单

交集:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *createList(int arr[], int len) {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
Node *p = head;
for (int i = 0; i < len; i++) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
p->next = newNode;
p = p->next;
}
return head;
}
Node *getIntersection(Node *list1, Node *list2) {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
Node *p = head;
Node *p1 = list1->next;
while (p1 != NULL) {
Node *p2 = list2->next;
while (p2 != NULL) {
if (p1->data == p2->data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = p1->data;
newNode->next = NULL;
p->next = newNode;
p = p->next;
break;
}
p2 = p2->next;
}
p1 = p1->next;
}
return head;
}
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[] = {3, 4, 5, 6, 7};
Node *list1 = createList(arr1, 5);
Node *list2 = createList(arr2, 5);
Node *intersection = getIntersection(list1, list2);
Node *p = intersection->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
```
并集:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
Node *createList(int arr[], int len) {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
Node *p = head;
for (int i = 0; i < len; i++) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = NULL;
p->next = newNode;
p = p->next;
}
return head;
}
Node *getUnion(Node *list1, Node *list2) {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
Node *p = head;
Node *p1 = list1->next;
while (p1 != NULL) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = p1->data;
newNode->next = NULL;
p->next = newNode;
p = p->next;
p1 = p1->next;
}
Node *p2 = list2->next;
while (p2 != NULL) {
Node *p1 = list1->next;
int flag = 0;
while (p1 != NULL) {
if (p2->data == p1->data) {
flag = 1;
break;
}
p1 = p1->next;
}
if (!flag) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = p2->data;
newNode->next = NULL;
p->next = newNode;
p = p->next;
}
p2 = p2->next;
}
return head;
}
int main() {
int arr1[] = {1, 2, 3, 4, 5};
int arr2[] = {3, 4, 5, 6, 7};
Node *list1 = createList(arr1, 5);
Node *list2 = createList(arr2, 5);
Node *unionList = getUnion(list1, list2);
Node *p = unionList->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
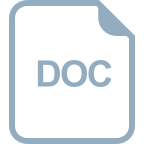


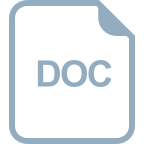
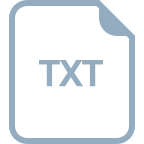
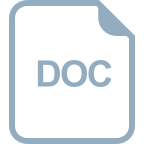
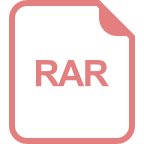
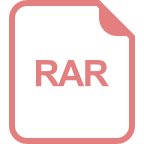
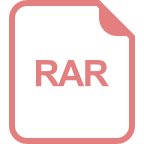
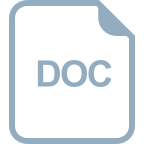
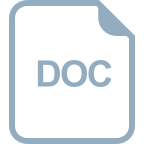
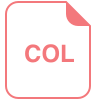
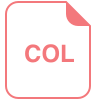
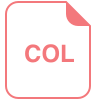
