def backward(ctx, grad_x, grad_geometry): (mask,) = ctx.saved_tensors # Since the operation is summing, we simply need to send gradient # to all elements that were part of the summation process.为什么反向传播需要mask
时间: 2024-03-31 20:32:44 浏览: 48
在 `VoxelsSumming` 中,`mask` 是在前向传播中根据输入几何信息计算出来的一个掩码张量,用于标记哪些像素需要参与求和,哪些像素不需要参与。在前向传播中,`mask` 被用于将输入张量 `x` 中的不需要参与求和的像素设置为0。
在反向传播中,由于前向传播是一个求和操作,因此需要将输出张量对输入张量 `x` 的梯度传递到所有参与了求和的像素上,而不需要传递到不参与求和的像素上。为了实现这个过程,需要使用 `mask` 对梯度进行过滤,只保留参与求和的像素的梯度。具体来说,就是将梯度张量 `grad_x` 与掩码张量 `mask` 相乘,得到只包含参与求和的像素梯度的张量。这样,就能够实现梯度仅传递到参与求和的像素上的效果。
因此,在 `backward` 方法中,需要使用 `mask` 对梯度进行过滤,以确保梯度仅传递到参与求和的像素上。
相关问题
class AveSupPixPoolFunction(torch.autograd.Function): @staticmethod def forward(ctx, img, spx): spx = spx.to(torch.int) K = spx.max()+1 assert(spx.size()[-2:]==img.size()[-2:]) out = spx_gpu.ave_forward(img, spx, K) outputs, pool_size = out outputs /= pool_size.to(torch.float) ctx.save_for_backward(pool_size, img, spx, K) return outputs @staticmethod def backward(ctx, grad_output): pool_size, img, spx, K = ctx.saved_tensors grad_input = grad_output / pool_size.to(torch.float) grad_input = SupPixUnpool()(grad_input, spx.long()) return grad_input, torch.zeros_like(spx) class AveSupPixPool(torch.nn.Module): def __init__(self): super(AveSupPixPool, self).__init__() def forward(self, img, spx): return AveSupPixPoolFunction.apply(img, spx)
这是一个 PyTorch 中自定义的超像素平均池化函数及其对应的模块。
该函数实现了超像素平均池化操作,输入参数为原始图像和超像素分割结果,返回值为池化后的结果。
其中,`forward` 函数实现了前向传播,使用了 PyTorch 的自动求导机制;`backward` 函数实现了后向传播,即求解梯度。
`AveSupPixPool` 类将 `AveSupPixPoolFunction` 封装成了 PyTorch 的模块,方便调用。
需要注意的是,该代码中使用了 `spx_gpu.ave_forward` 函数,这是一个自定义的 CUDA 函数,用于在 GPU 上进行超像素平均池化操作。如果要使用该代码,需要先编译 `spx_gpu.ave_forward` 函数并加载到 PyTorch 中。
另外,该代码中的超像素平均池化操作是基于超像素分割结果进行操作的,如果没有进行超像素分割,需要先对原始图像进行超像素分割操作,然后再使用该函数进行池化操作。
class GradientDecoupleLayer(Function): @staticmethod def forward(ctx, x, _lambda): ctx._lambda = _lambda return x @staticmethod def backward(ctx, grad_output): grad_output = grad_output * ctx._lambda return grad_output, None class AffineLayer(nn.Module): def __init__(self, num_channels, bias=False): super(AffineLayer, self).__init__() weight = torch.FloatTensor(1, num_channels, 1, 1).fill_(1) self.weight = nn.Parameter(weight, requires_grad=True) self.bias = None if bias: bias = torch.FloatTensor(1, num_channels, 1, 1).fill_(0) self.bias = nn.Parameter(bias, requires_grad=True) def forward(self, X): out = X * self.weight.expand_as(X) if self.bias is not None: out = out + self.bias.expand_as(X) return out def decouple_layer(x, _lambda): return GradientDecoupleLayer.apply(x, _lambda)
这段代码看起来是 PyTorch 的代码,其中定义了两个类:GradientDecoupleLayer 和 AffineLayer。GradientDecoupleLayer 是一个自定义的 PyTorch 函数,实现了一个梯度解耦的功能;AffineLayer 是一个继承自 nn.Module 的类,实现了一个仿射变换的功能。其中,AffineLayer 的实现比较简单,就是将输入 X 乘上一个可学习的权重参数 self.weight,再加上一个可选的偏置参数 self.bias;而 GradientDecoupleLayer 则是比较复杂的一个函数,它的作用是将输入 x 的梯度乘上一个常数 _lambda,然后输出。这个函数的实现用到了 PyTorch 的静态方法 apply,并且在 backward 函数中返回了梯度 grad_output 乘上 _lambda 的结果。
阅读全文
相关推荐
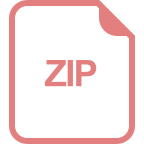
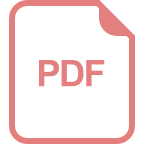
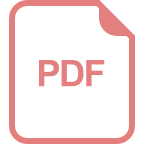











