class NeuralNetwork: def __init__(self, input_dim, hidden_dim, output_dim): self.input_dim = input_dim self.hidden_dim = hidden_dim self.output_dim = output_dim self.weights1 = np.random.randn(input_dim, hidden_dim) self.bias1 = np.zeros((1, hidden_dim)) self.weights2 = np.random.randn(hidden_dim, output_dim) self.bias2 = np.zeros((1, output_dim)) def relu(self, x): return np.maximum(0, x) def relu_derivative(self, x): return np.where(x >= 0, 1, 0) def forward(self, x): self.z1 = np.dot(x, self.weights1) + self.bias1 self.a1 = self.relu(self.z1) self.z2 = np.dot(self.a1, self.weights2) + self.bias2 self.y_hat = self.z2 return self.y_hat def backward(self, x, y, learning_rate): error = self.y_hat - y delta2 = error delta1 = np.dot(delta2, self.weights2.T) * self.relu_derivative(self.a1) grad_weights2 = np.dot(self.a1.T, delta2) grad_bias2 = np.sum(delta2, axis=0, keepdims=True) grad_weights1 = np.dot(x.T, delta1) grad_bias1 = np.sum(delta1, axis=0) self.weights2 -= learning_rate * grad_weights2 self.bias2 -= learning_rate * grad_bias2 self.weights1 -= learning_rate * grad_weights1 根据代码加上损失函数
时间: 2023-12-26 22:04:54 浏览: 106
好的,如果要加上损失函数的话,可以在backward方法中计算损失函数对网络输出的导数,然后将其与之前计算得到的delta2相乘得到最终的delta2。常见的损失函数有均方误差(MSE)和交叉熵(Cross Entropy)等,下面以MSE为例:
```
def mse_loss_derivative(self, y):
return 2*(self.y_hat - y) / self.output_dim
def backward(self, x, y, learning_rate):
error = self.y_hat - y
delta2 = self.mse_loss_derivative(y) * self.relu_derivative(self.z2) # 加上损失函数的导数
delta1 = np.dot(delta2, self.weights2.T) * self.relu_derivative(self.a1)
grad_weights2 = np.dot(self.a1.T, delta2)
grad_bias2 = np.sum(delta2, axis=0, keepdims=True)
grad_weights1 = np.dot(x.T, delta1)
grad_bias1 = np.sum(delta1, axis=0)
self.weights2 -= learning_rate * grad_weights2
self.bias2 -= learning_rate * grad_bias2
self.weights1 -= learning_rate * grad_weights1
self.bias1 -= learning_rate * grad_bias1
```
在这里,mse_loss_derivative方法计算了MSE损失函数对网络输出的导数,然后在计算delta2时将其与ReLU函数的导数相乘得到最终的delta2。接下来,该方法与之前的backward方法相同,计算梯度并更新参数。
阅读全文
相关推荐
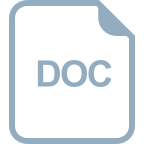
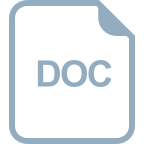
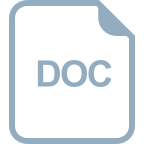

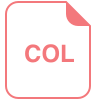
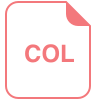
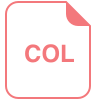
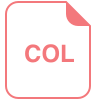
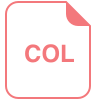
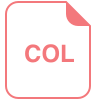
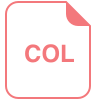
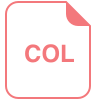
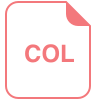
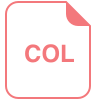
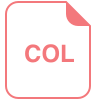
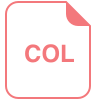


