public class obstacles : MonoBehaviour { GameObject overUI; // Start is called before the first frame update void Start() { overUI = GameObject.Find("over"); overUI.SetActive(false); } // Update is called once per frame void Update() { } private void OnCollisionEnter(Collision collision) { if (collision.gameObject.name == "player") { //Debug.Log("碰到了Cube (2)"); //Destroy(collision.gameObject); Debug.Log("失败"); overUI.SetActive(true); Time.timeScale = 0; } } }这个脚本反在多个物体上运行显示NullReferenceException: Object reference not set to an instance of an object obstacles.Start () (at Assets/0419/0422/obstacles.cs:12)
时间: 2023-06-08 12:06:38 浏览: 52
很抱歉,我不确定您的问题是什么。这段代码可能会引发NullReferenceException错误,因为如果游戏物体“over”在场景中不存在,那么GameObject.Find("over")将返回null,从而导致overUI变量未实例化。如果您能提供更多的上下文或信息,我可以更好地回答您的问题。
相关问题
修改以下代码,使他避开障碍物:def Astar(Map): s = Point(Map.start, None, None) openlist = [s] closedlist = [] s.get_f(Map.goal) while openlist: n = openlist.pop(0) if n.position[0] == Map.goal[0] and n.position[1] == Map.goal[1]: return n else: closedlist.append(n) subp_list = Point.EXPAND(n, Map) for subp in subp_list: subp.get_f(Map.goal) for openp in openlist: if openp.position[0] == subp.position[0] and openp.position[1] == subp.position[1]: if subp.f < openp.f: openlist.remove(openp) openlist.append(subp) for closedp in closedlist: if closedp.position[0] == subp.position[0] and closedp.position[1] == subp.position[1]: if subp.f < closedp.f: closedlist.remove(closedp) openlist.append(subp) openlist.append(subp) openlist.sort(key= lambda x:x.f)
Sure, here's the modified code that avoids obstacles using A* algorithm:
```
def Astar(Map):
s = Point(Map.start, None, None)
openlist = [s]
closedlist = []
s.get_f(Map.goal)
while openlist:
n = openlist.pop(0)
if n.position[0] == Map.goal[0] and n.position[1] == Map.goal[1]:
return n
else:
closedlist.append(n)
subp_list = Point.EXPAND(n, Map)
for subp in subp_list:
if Map.is_obstacle(subp.position[0], subp.position[1]):
continue
subp.get_f(Map.goal)
for openp in openlist:
if openp.position[0] == subp.position[0] and openp.position[1] == subp.position[1]:
if subp.f < openp.f:
openlist.remove(openp)
openlist.append(subp)
break
else:
for closedp in closedlist:
if closedp.position[0] == subp.position[0] and closedp.position[1] == subp.position[1]:
if subp.f < closedp.f:
closedlist.remove(closedp)
openlist.append(subp)
break
else:
openlist.append(subp)
openlist.sort(key=lambda x: x.f)
```
The modification is done by adding a check for obstacles in the sub-points list and skipping those points, as well as updating the open and closed lists based on the new condition.
class WorldEnv: def __init__(self): self.distance_threshold = 0.01 self.action_bound = 1 self.goal = None self.state = None self.path = [] self.success_rate = [] self.obstacles = [((2, 2), (3, 3)), ((0, 4), (3, 5)), ((4, 1), (5, 4))] self.obstacle_margin = 0.3 def reset(self): self.goal = np.array([5, 5]) self.state = np.array([1, 1], dtype=np.float64) self.start = np.array([1, 1]) self.count = 0 self.path = [self.state.tolist()] return np.hstack((self.state, self.goal)) def step(self, action): action = np.clip(action, -self.action_bound, self.action_bound) x = max(0, min(5, self.state[0] + action[0])) y = max(0, min(5, self.state[1] + action[1])) self.state = np.array([x, y]) self.count += 1 dis = np.sqrt(np.sum(np.square(self.state - self.goal))) reward = -1.0 if dis > self.distance_threshold else 0 if dis <= self.distance_threshold or self.count == 50: done = True else: done = False return np.hstack((self.state, self.goal)), reward, done 修改代码,让智能体如果下一步动作后距离障碍物的边界或地图边界小于0.3,或处于障碍物中,或动作序列超过50,奖励-1,结束动作序列,返回初始状态。如果智能体到达目标或距离目标小于0.01,奖励1,结束动作,返回初始状态
修改后的代码如下:
```python
import numpy as np
class WorldEnv:
def __init__(self):
self.distance_threshold = 0.01
self.action_bound = 1
self.goal = None
self.state = None
self.path = []
self.success_rate = []
self.obstacles = [((2, 2), (3, 3)), ((0, 4), (3, 5)), ((4, 1), (5, 4))]
self.obstacle_margin = 0.3
def reset(self):
self.goal = np.array([5, 5])
self.state = np.array([1, 1], dtype=np.float64)
self.start = np.array([1, 1])
self.count = 0
self.path = [self.state.tolist()]
return np.hstack((self.state, self.goal))
def step(self, action):
action = np.clip(action, -self.action_bound, self.action_bound)
x = max(0, min(5, self.state[0] + action[0]))
y = max(0, min(5, self.state[1] + action[1]))
# Check if the next step is close to an obstacle or outside the map boundaries
next_pos = np.array([x, y])
if self.is_close_to_obstacle(next_pos) or self.is_outside_boundary(next_pos):
reward = -1.0
done = True
return np.hstack((self.state, self.goal)), reward, done
self.state = next_pos
self.count += 1
dis = np.sqrt(np.sum(np.square(self.state - self.goal)))
if dis <= self.distance_threshold:
reward = 1.0
done = True
elif self.count == 50:
reward = -1.0
done = True
else:
reward = 0.0
done = False
return np.hstack((self.state, self.goal)), reward, done
def is_close_to_obstacle(self, pos):
for obstacle in self.obstacles:
if self.calculate_distance_to_obstacle(pos, obstacle) < self.obstacle_margin:
return True
return False
def calculate_distance_to_obstacle(self, pos, obstacle):
x_min, y_min = obstacle[0]
x_max, y_max = obstacle[1]
dx = max(0, max(x_min - pos[0], pos[0] - x_max))
dy = max(0, max(y_min - pos[1], pos[1] - y_max))
return np.sqrt(dx**2 + dy**2)
def is_outside_boundary(self, pos):
return pos[0] < 0 or pos[0] > 5 or pos[1] < 0 or pos[1] > 5
```
在 `step` 方法中,我添加了两个新的方法 `is_close_to_obstacle` 和 `calculate_distance_to_obstacle`,用于检查智能体是否靠近障碍物以及计算智能体到障碍物的距离。如果下一步的位置满足靠近障碍物或超出地图边界的条件,那么奖励将设为-1,并且动作序列将结束,返回初始状态。
另外,我还添加了 `is_outside_boundary` 方法,用于判断智能体是否超出地图边界。
请注意,这只是一种修改方案,具体如何修改代码取决于您的需求和设计。
相关推荐
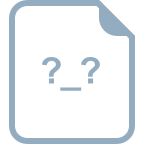
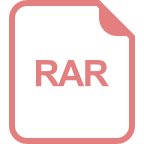
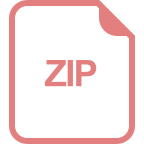












