如何用python利用形态滤波找到图片中的椭圆并随机在椭圆轮廓上随机提取八个点的坐标
时间: 2024-03-23 14:43:53 浏览: 68
可以使用OpenCV库来实现形态滤波和椭圆检测,并使用随机数生成器来随机选择椭圆上的点。
以下是一个示例代码,用于在图像中检测椭圆并随机选择椭圆轮廓上的八个点:
```python
import cv2
import random
# 读取图片
img = cv2.imread('image.jpg')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行形态学操作,以便增强椭圆的检测
kernel = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (3, 3))
gray = cv2.morphologyEx(gray, cv2.MORPH_GRADIENT, kernel)
# 检测椭圆
contours, hierarchy = cv2.findContours(gray, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
ellipses = []
for cnt in contours:
if cnt.shape[0] < 5:
continue
ellipse = cv2.fitEllipse(cnt)
ellipses.append(ellipse)
# 随机选择一个椭圆
ellipse = random.choice(ellipses)
# 在椭圆轮廓上随机选择八个点
points = cv2.ellipse2Poly(ellipse, -1)
random_points = random.sample(list(points), 8)
# 显示结果
cv2.drawContours(img, [points], 0, (0, 255, 0), 2)
for point in random_points:
cv2.circle(img, tuple(point), 3, (0, 0, 255), -1)
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,这只是一个简单的示例代码,需要根据实际需求进行修改和适当的优化。
阅读全文
相关推荐
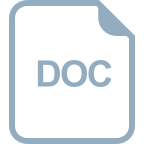
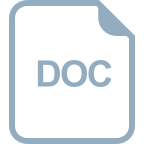
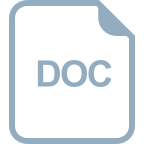

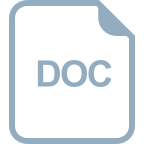
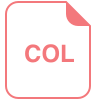
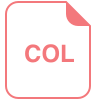
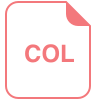
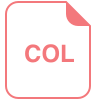
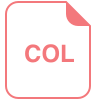
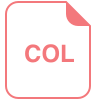
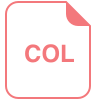
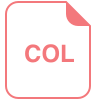
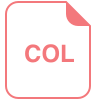
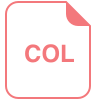
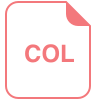
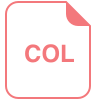
