用Java写一段代码,给定一个时间字段deadline(yyyyMMdd),判断是今天或昨天或昨天之前的
时间: 2024-03-24 14:41:10 浏览: 84
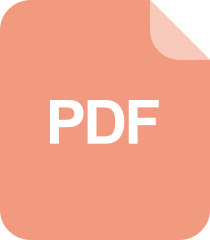
JS获取当前时间的实例代码(昨天、今天、明天)
好的,以下是Java代码实现:
```java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class DeadlineChecker {
public static void main(String[] args) {
String deadline = "20220101"; // 设定截止日期
String result = checkDeadline(deadline);
System.out.println(result);
}
public static String checkDeadline(String deadline) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
Calendar deadlineCal = Calendar.getInstance();
try {
Date date = sdf.parse(deadline);
deadlineCal.setTime(date);
} catch (ParseException e) {
e.printStackTrace();
}
Calendar now = Calendar.getInstance();
now.setTime(new Date());
if (deadlineCal.get(Calendar.YEAR) == now.get(Calendar.YEAR)
&& deadlineCal.get(Calendar.MONTH) == now.get(Calendar.MONTH)
&& deadlineCal.get(Calendar.DAY_OF_MONTH) == now.get(Calendar.DAY_OF_MONTH)) {
return "今天";
} else {
now.add(Calendar.DAY_OF_MONTH, -1);
if (deadlineCal.get(Calendar.YEAR) == now.get(Calendar.YEAR)
&& deadlineCal.get(Calendar.MONTH) == now.get(Calendar.MONTH)
&& deadlineCal.get(Calendar.DAY_OF_MONTH) == now.get(Calendar.DAY_OF_MONTH)) {
return "昨天";
} else {
now.add(Calendar.DAY_OF_MONTH, -1);
if (deadlineCal.before(now)) {
return "昨天之前";
}
}
}
return "未知";
}
}
```
这个代码和上面的代码很相似,只不过为了支持yyyyMMdd格式的日期,我们修改了 `SimpleDateFormat` 的模板为 `"yyyyMMdd"`,并调用 `Calendar` 对象的 `get(Calendar.DAY_OF_MONTH)` 方法来获取日期。其他逻辑和上面的代码一样,不再赘述。
同样的,我们也设定的截止日期为 `20220101`,根据当前时间的不同,输出结果可能为 `今天`、`昨天`或`昨天之前`。
阅读全文
相关推荐






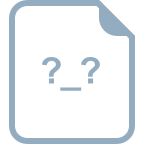
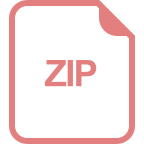







