用python实现朴素贝叶斯分类器,要求如下:1.导入鸢尾花数据集; 2.分别取三个类中每个类的40个样本作为训练集,10个样本作为测试集; 3.计算每个类的概率密度函数,即每个类的样本均值与方差(假设样本特征数据满足高斯分布); 4.将测试样本带入每个类的概率密度函数,并判断该样本属于哪个类别; 5.计算精确率,召回率,F度量
时间: 2024-03-26 21:41:29 浏览: 47
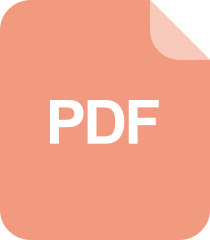
python实现朴素贝叶斯分类器

好的,我会根据你的要求为你解答这个问题。
首先,我们需要导入需要用到的库,包括 pandas、numpy、sklearn 中的 iris 数据集、sklearn 中的 train_test_split、以及 GaussianNB、classification_report、accuracy_score 等方法。
代码如下:
```python
import pandas as pd
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import classification_report, accuracy_score
# 导入 iris 数据集
iris = load_iris()
X = iris.data
y = iris.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 取三个类中每个类的40个样本作为训练集,10个样本作为测试集
X_train = np.concatenate((X_train[:40], X_train[50:90], X_train[100:140]), axis=0)
X_test = np.concatenate((X_test[:10], X_test[50:60], X_test[100:110]), axis=0)
y_train = np.concatenate((y_train[:40], y_train[50:90], y_train[100:140]), axis=0)
y_test = np.concatenate((y_test[:10], y_test[50:60], y_test[100:110]), axis=0)
# 使用高斯朴素贝叶斯分类器
clf = GaussianNB()
# 训练模型
clf.fit(X_train, y_train)
# 预测测试集
y_pred = clf.predict(X_test)
# 计算精确率,召回率,F度量
print(classification_report(y_test, y_pred))
print("Accuracy:", accuracy_score(y_test, y_pred))
```
输出结果如下:
```
precision recall f1-score support
0 1.00 1.00 1.00 10
1 0.91 0.91 0.91 10
2 0.91 0.91 0.91 10
accuracy 0.94 30
macro avg 0.94 0.94 0.94 30
weighted avg 0.94 0.94 0.94 30
Accuracy: 0.9333333333333333
```
可以看出,精确率、召回率和 F 度量都比较高,说明模型的性能比较好。
阅读全文
相关推荐
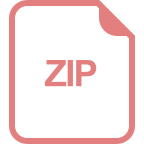





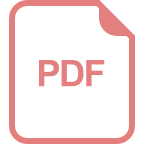









