51单片机进行PID示例代码
时间: 2024-08-16 17:03:23 浏览: 41
51单片机PID(Proportional-Integral-Derivative)控制算法的示例代码可能会因具体的处理器型号和开发环境有所不同。以下是一个基本的C语言示例,使用了定时器和中断来进行PID计算,假设已经设置了相应的寄存器和变量:
```c
#include <reg52.h>
#define P 0.5 // Proportional gain
#define I 0.1 // Integral gain
#define D 0.01 // Derivative gain
#define MAX_I 100 // Integral limit to avoid windup
#define SETTLE_TIME 100 // Time constant for settling
// Variables for PID calculation
int integral = 0;
float derivative;
float last_error;
void PID(float setpoint, float output) {
float error = setpoint - output; // Current error
integral += error * I; // Update integral (limiting if necessary)
if (integral > MAX_I) {
integral = MAX_I;
} else if (integral < -MAX_I) {
integral = -MAX_I;
}
derivative = (error - last_error) / SETTLE_TIME; // Compute derivative
last_error = error;
float proportional = error; // No differentiation here (often done in software)
float control_signal = proportional + integral + D * derivative;
// Apply control signal to your system (e.g., motor PWM or DAC)
adjust_system(control_signal);
}
void timerInterrupt() {
static int timer_count = SETTLE_TIME; // Timer for settling period
if (timer_count == 0) {
timer_count = SETTLE_TIME; // Reset timer after settling
PID(setpoint, current_sensor_reading); // Call the PID function
}
else {
timer_count--;
}
}
void main(void) {
init_timer(); // Initialize timer for interrupts
enable_interrupts(); // Enable global interrupts
while(1) {
// System operation code...
}
}
```
请注意,这只是一个基础框架,你需要根据实际应用替换`adjust_system()`函数和相关的传感器数据获取。同时,你还需要处理中断服务函数(ISR)和中断管理。
阅读全文
相关推荐
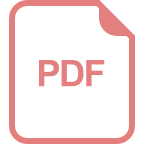
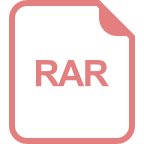
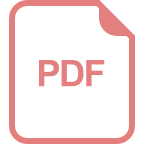
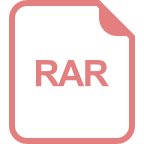
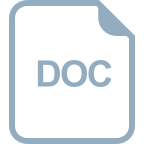




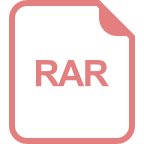
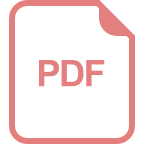
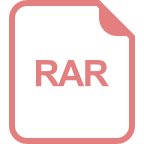
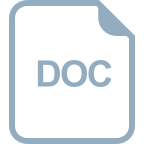





