unity 写一个在有蓝牙模块的windows设备上运行的可以搜索蓝牙的代码
时间: 2024-02-01 17:17:10 浏览: 21
以下是一个简单的Unity蓝牙搜索代码示例:
```csharp
using UnityEngine;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
using System.Threading.Tasks;
public class BluetoothScanner : MonoBehaviour
{
private List<string> _devices = new List<string>();
[DllImport("BluetoothAPI")]
private static extern bool BluetoothFindFirstDevice(out System.IntPtr handle, ref BLUETOOTH_DEVICE_SEARCH_PARAMS searchParams, ref BLUETOOTH_DEVICE_INFO deviceInfo);
[DllImport("BluetoothAPI")]
private static extern bool BluetoothFindNextDevice(System.IntPtr hFind, ref BLUETOOTH_DEVICE_INFO deviceInfo);
[DllImport("BluetoothAPI")]
private static extern bool BluetoothFindDeviceClose(System.IntPtr hFind);
[StructLayout(LayoutKind.Sequential)]
private struct BLUETOOTH_DEVICE_SEARCH_PARAMS
{
public int dwSize;
public bool fReturnAuthenticated;
public bool fReturnRemembered;
public bool fReturnUnknown;
public bool fReturnConnected;
public bool fIssueInquiry;
public byte cTimeoutMultiplier;
public System.IntPtr hRadio;
}
[StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)]
private struct BLUETOOTH_DEVICE_INFO
{
public int dwSize;
public long Address;
public uint ulClassofDevice;
[MarshalAs(UnmanagedType.Bool)]
public bool fConnected;
[MarshalAs(UnmanagedType.Bool)]
public bool fRemembered;
[MarshalAs(UnmanagedType.Bool)]
public bool fAuthenticated;
public SYSTEMTIME stLastSeen;
public SYSTEMTIME stLastUsed;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 248)]
public string szName;
}
[StructLayout(LayoutKind.Sequential)]
private struct SYSTEMTIME
{
public ushort wYear;
public ushort wMonth;
public ushort wDayOfWeek;
public ushort wDay;
public ushort wHour;
public ushort wMinute;
public ushort wSecond;
public ushort wMilliseconds;
}
private async void Start()
{
await Task.Delay(1000); // 等待一秒钟,让蓝牙模块初始化
BLUETOOTH_DEVICE_SEARCH_PARAMS searchParams = new BLUETOOTH_DEVICE_SEARCH_PARAMS();
searchParams.dwSize = Marshal.SizeOf(typeof(BLUETOOTH_DEVICE_SEARCH_PARAMS));
searchParams.fReturnAuthenticated = true;
searchParams.fReturnRemembered = true;
searchParams.fReturnUnknown = true;
searchParams.fReturnConnected = true;
searchParams.cTimeoutMultiplier = 10;
System.IntPtr searchHandle;
BLUETOOTH_DEVICE_INFO deviceInfo = new BLUETOOTH_DEVICE_INFO();
deviceInfo.dwSize = Marshal.SizeOf(typeof(BLUETOOTH_DEVICE_INFO));
if (BluetoothFindFirstDevice(out searchHandle, ref searchParams, ref deviceInfo))
{
do
{
_devices.Add(deviceInfo.szName);
}
while (BluetoothFindNextDevice(searchHandle, ref deviceInfo));
}
BluetoothFindDeviceClose(searchHandle);
Debug.Log("Found " + _devices.Count + " Bluetooth devices:");
foreach (string device in _devices)
{
Debug.Log(device);
}
}
}
```
上述代码使用了Windows API函数来搜索Bluetooth设备。它可以在Unity中使用,但是需要编译为Windows应用程序才能运行,因为它使用Windows API函数来访问蓝牙硬件。
相关推荐
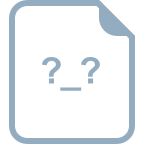
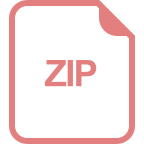














