The method readObject() is undefined for the type Student1
时间: 2024-03-02 10:50:34 浏览: 135
这个错误通常发生在Java中,意味着您正在尝试使用一个没有定义在Student1类中的readObject()方法。readObject()方法是用于反序列化对象的方法,如果您想要在Student1类中使用它,您需要在类中定义它。您可以尝试添加以下代码:
```
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
// add your code here
}
```
请注意,您需要根据您的需求编写方法体。另外,如果您不需要在Student1类中使用readObject()方法,您可以检查代码中是否有意外的调用该方法,并将其删除。
相关问题
对这段代码进行改错import java.io.*; import java.util.*; public class Test { public static void main(String[] args) throws IOException, ClassNotFoundException { List<Student> students = new ArrayList<>(); students.add(new Student("001", "张三", 90)); students.add(new Student("002", "李四", 80)); students.add(new Student("003", "王五", 85)); students.add(new Student("004", "赵六", 95)); // 排序并保存到文件 Collections.sort(students); ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("stud.dat")); out.writeObject(students); out.close(); // 从文件读取并显示学生信息 ObjectInputStream in = new ObjectInputStream(new FileInputStream("stud.dat")); List<Student> sortedStudents = (List<Student>) in.readObject(); for (Student student : sortedStudents) { System.out.println(student); } in.close(); } }
这段代码本身没有错误,但是需要注意以下几点:
1. `Student`类必须实现`Serializable`接口,以便可以使用`ObjectOutputStream`将对象序列化到文件中,和使用`ObjectInputStream`从文件中反序列化对象。
2. 如果文件`stud.dat`不存在,则会抛出`FileNotFoundException`异常。这时可以考虑在程序中创建文件,或者在异常处理中进行处理。
3. 对于排序过程,如果`Student`类没有实现`Comparable`接口,则需要提供一个比较器`Comparator`作为参数传入`Collections.sort()`方法中。
希望这些注意点能够帮助你更好地理解和运行这段代码。
Serializability of a class is enabled by the class implementing the java.io.Serializable interface. Classes that do not implement this interface will not have any of their state serialized or deserialized. All subtypes of a serializable class are themselves serializable. The serialization interface has no methods or fields and serves only to identify the semantics of being serializable. To allow subtypes of non-serializable classes to be serialized, the subtype may assume responsibility for saving and restoring the state of the supertype's public, protected, and (if accessible) package fields. The subtype may assume this responsibility only if the class it extends has an accessible no-arg constructor to initialize the class's state. It is an error to declare a class Serializable if this is not the case. The error will be detected at runtime. During deserialization, the fields of non-serializable classes will be initialized using the public or protected no-arg constructor of the class. A no-arg constructor must be accessible to the subclass that is serializable. The fields of serializable subclasses will be restored from the stream. When traversing a graph, an object may be encountered that does not support the Serializable interface. In this case the NotSerializableException will be thrown and will identify the class of the non-serializable object. Classes that require special handling during the serialization and deserialization process must implement special methods with these exact signatures: private void writeObject(java.io.ObjectOutputStream out) throws IOException private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException; private void readObjectNoData() throws ObjectStreamException;
一个类实现了java.io.Serializable接口就可以启用类的序列化功能。没有实现这个接口的类将不会序列化或反序列化其任何状态。可序列化类的所有子类型也都是可序列化的。序列化接口没有方法或字段,仅用于标识可序列化的语义。
为了允许非可序列化类的子类型被序列化,子类型可以承担起保存和恢复超类型的公共、受保护和(如果可访问)包字段状态的责任。只有当扩展的类具有可访问的无参构造函数来初始化类的状态时,子类型才能承担这个责任。如果不满足这个条件,声明一个类为Serializable将会导致错误,在运行时将会被检测到。
在反序列化过程中,非可序列化类的字段将使用该类的公共或受保护的无参构造函数进行初始化。无参构造函数必须对可序列化的子类可访问。可序列化子类的字段将从流中恢复。
在遍历图形时,可能会遇到不支持Serializable接口的对象。在这种情况下,将抛出NotSerializableException,并且会标识非可序列化对象的类。
需要在序列化和反序列化过程中进行特殊处理的类必须实现具有以下确切签名的特殊方法:
private void writeObject(java.io.ObjectOutputStream out)
throws IOException
private void readObject(java.io.ObjectInputStream in)
throws IOException, ClassNotFoundException;
private void readObjectNoData()
throws ObjectStreamException;
阅读全文
相关推荐
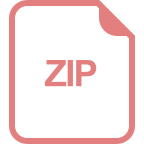
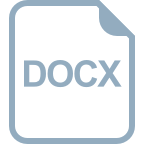













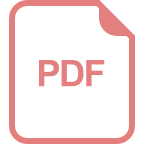