实现链队的各种基本运算的算法
时间: 2023-06-09 17:08:16 浏览: 209
链队的基本运算包括入队(enqueue)、出队(dequeue)、获取队首元素(getFront)、获取队尾元素(getRear)、判断队列是否为空(isEmpty)等。具体算法如下:
1. 入队(enqueue)算法:
a. 创建一个新结点;
b. 将新结点的值赋为待插入的元素;
c. 如果队列为空,则将队首和队尾指针都指向新结点,否则将当前队尾结点的next指针指向新结点,再将队尾指针指向新结点。
2. 出队(dequeue)算法:
a. 如果队列为空,则返回空值;
b. 否则,取出队首结点的元素值,将队首指针指向下一个结点,最后释放原队首结点的内存空间。
3. 获取队首元素(getFront)算法:
a. 如果队列为空,则返回空值;
b. 否则,返回队首结点的元素值。
4. 获取队尾元素(getRear)算法:
a. 如果队列为空,则返回空值;
b. 否则,返回队尾结点的元素值。
5. 判断队列是否为空(isEmpty)算法:
a. 如果队列为空,则返回真(true);
b. 否则,返回假(false)。
以上就是链队的各种基本运算的算法实现。
相关问题
实验题4实现链队的各种基本运算的算法 目的:领会链队的存储结构和掌握链队中各种基本运算算法的设计。 内容:编写一个程序liqueue.cpp,实现链队(假设栈中的元素类型ElemType 为 char
链队是一种基于链表的数据结构,它维护了一个先进先出(First In First Out,FIFO)的原则。在实现链队的多种基本运算时,主要包括入队 Enqueue、出队 Dequeue 和查看队头 Peek 等操作。对于实验题目 4,你需要完成以下功能:
1. **入队 (Enqueue)**: 当需要添加一个新元素到队列尾部时,创建一个新的节点并将其链接到当前队尾节点之后。
```cpp
void enqueue(ElemType data) {
Node* newNode = new Node(data);
if (!head) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
```
2. **出队 (Dequeue)**: 移除并返回队头的第一个元素,同时更新队头指针。如果队列为空,处理特殊情况(例如抛出异常)。
```cpp
void dequeue() {
if (empty()) {
throw EmptyQueueException();
} else {
ElemType data = head->data;
Node* temp = head;
head = head->next;
delete temp;
if (head == NULL) {
tail = NULL;
}
}
}
```
3. **查看队头 (Peek or Front)**: 返回队头元素,但不移除它,用于检查队列的内容而不想改变其顺序。
```cpp
ElemType peek() const {
if (empty()) {
throw EmptyQueueException();
}
return head->data;
}
```
4. **判断空队 (IsEmpty)**: 检查队列是否为空。
```cpp
bool empty() const {
return head == NULL;
}
```
5. **获取队列长度 (Size)**: 计算队列中有多少元素。
```cpp
int size() const {
int count = 0;
Node* current = head;
while (current != NULL) {
++count;
current = current->next;
}
return count;
}
```
在这个`liqueue.cpp`文件中,你需要将上述函数组织起来,并确保在使用过程中能够正确处理边界条件和内存管理。记得在适当的地方包含所需的头文件以及处理错误的情况。如果你有其他关于这个任务的具体疑问,请随时提问。
实现链串(LiString.cpp)中各种基本运算的算法C++编程代码
在`LiString.cpp`中,实现链串的基本运算通常包括插入、删除、查找、连接等操作。这里是一个简单的示例代码,展示了如何在C++中使用链表结构实现字符串:
```cpp
// 定义节点类
class Node {
public:
char data;
Node* next;
Node(char c) : data(c), next(nullptr) {}
};
// 链串类
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
// 插入字符到末尾
void insert(char c) {
if (!head) {
head = new Node(c);
return;
}
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node(c);
}
// 删除指定位置的字符
void remove(int index) {
if (!head || index < 0 || index >= size()) {
return;
}
if (index == 0) {
Node* temp = head;
head = head->next;
delete temp;
} else {
Node* current = head;
for (int i = 0; i < index - 1; ++i) {
current = current->next;
}
Node* toRemove = current->next;
current->next = toRemove->next;
delete toRemove;
}
}
// 查找指定字符
bool find(char c) const {
Node* current = head;
while (current) {
if (current->data == c) {
return true;
}
current = current->next;
}
return false;
}
// 连接两个链串
LinkedList concatenate(const LinkedList& other) {
if (!head) {
head = other.head;
return *this;
}
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = other.head;
return *this;
}
// 获取链串长度
int size() const {
int count = 0;
Node* current = head;
while (current) {
count++;
current = current->next;
}
return count;
}
};
```
阅读全文
相关推荐
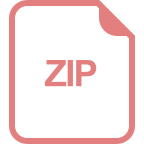
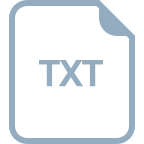
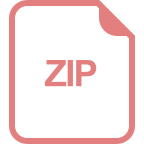













