flask yolov5 code
时间: 2023-07-21 22:59:08 浏览: 106
Sure! Here's an example code snippet showing how to integrate YOLOv5 with Flask:
```python
from flask import Flask, request, jsonify
from PIL import Image
import torch
app = Flask(__name__)
# Load YOLOv5 model
model = torch.hub.load('ultralytics/yolov5', 'yolov5s', pretrained=True).autoshape()
@app.route('/detect', methods=['POST'])
def detect_objects():
# Get image from request
image_file = request.files['image']
image = Image.open(image_file).convert('RGB')
# Perform object detection
results = model(image)
# Extract bounding box coordinates and class labels
boxes = results.xyxy[0].tolist()
labels = results.names[0]
# Prepare response
response = {
'boxes': boxes,
'labels': labels
}
return jsonify(response)
if __name__ == '__main__':
app.run()
```
This code sets up a Flask server that listens for POST requests on the `/detect` endpoint. It expects an image file in the request payload. The YOLOv5 model is loaded using `torch.hub.load()` and performs object detection on the input image. The detected bounding box coordinates and class labels are then returned as a JSON response.
Note: Make sure you have the necessary dependencies installed, such as Flask, PIL, and torch. You can install them using pip:
```
pip install flask pillow torch torchvision
```
Remember to customize and modify the code according to your specific requirements and file paths.
阅读全文
相关推荐
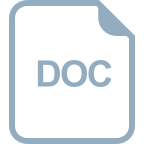
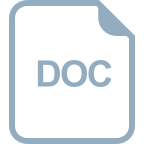
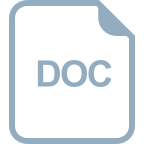
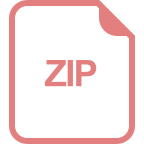
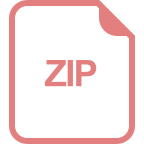
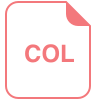


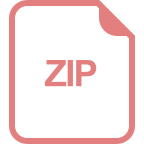






