(2)继承上题中的Person类,派生学生类Student,要求如下: Student类的成员变量: score 表示学生的成绩(整数类型),私有属性 Student类的方法成员: 构造方法 ,参数(self,name, sex,score) ,前两个参数调用父类方法设置 getScore (self) 获得学生的成绩 setScore (self,score) 获得学生的成绩,验证成绩在0-100之间有效 __str__(self)方法, 返回人的姓名和性别和成绩
时间: 2024-03-05 21:51:35 浏览: 24
以下是实现您所需的代码:
```python
class Student(Person):
def __init__(self, name, sex, score):
super().__init__(name, sex)
self.__score = score
def get_score(self):
return self.__score
def set_score(self, score):
if score >= 0 and score <= 100:
self.__score = score
else:
print('成绩输入错误')
def __str__(self):
return super().__str__() + ', 成绩:' + str(self.__score)
# 测试代码
student = Student('张三', 'M', 90)
print(student)
student.set_score(80)
print(student)
```
输出结果为:
```
姓名:张三, 性别:男, 成绩:90
姓名:张三, 性别:男, 成绩:80
```
相关问题
(1)根据下面的要求实现“人”类Person: Person类的属性: name 姓名 ,私有属性(注意私有属性的设置方式) sex 性别 ,私有属性 Person类的方法: 构造方法 ,有参数(self,name, sex) get_name(self) 获得人的姓名 get_sex(self) 获得人的性别,返回’男’,或‘女’ set_name(self,name) 设置姓名 set_sex(self,sex) 设置性别,只能接受’m’,’w’或’M’,’W’,表示男,女。 __str__(self)方法, 返回人的姓名和性别 (2)继承上题中的Person类,派生学生类Student,要求如下: Student类的成员变量: score 表示学生的成绩(整数类型),私有属性 Student类的方法成员: 构造方法 ,参数(self,name, sex,score) ,前两个参数调用父类方法设置 getScore (self) 获得学生的成绩 setScore (self,score) 获得学生的成绩,验证成绩在0-100之间有效 __str__(self)方法, 返回人的姓名和性别和成绩 (3)根据你上面的设计,若有语句: s0=Person(“wang”, “m”); print(s0) s1=Student(“lee”, “W”,90); print(s1.__str__()) 请写出上面程序段的运行结果。
代码实现如下:
```python
class Person:
def __init__(self, name, sex):
self.__name = name
self.__sex = sex
def get_name(self):
return self.__name
def get_sex(self):
return '男' if self.__sex == 'm' or self.__sex == 'M' else '女'
def set_name(self, name):
self.__name = name
def set_sex(self, sex):
if sex in ['m', 'M', 'w', 'W']:
self.__sex = sex
def __str__(self):
return f"姓名:{self.__name},性别:{self.get_sex()}"
class Student(Person):
def __init__(self, name, sex, score):
super().__init__(name, sex)
self.__score = score
def get_score(self):
return self.__score
def set_score(self, score):
if 0 <= score <= 100:
self.__score = score
def __str__(self):
return f"{super().__str__()},成绩:{self.__score}"
```
运行以下代码:
```python
s0 = Person("wang", "m")
print(s0)
s1 = Student("lee", "W", 90)
print(s1.__str__())
```
输出:
```
姓名:wang,性别:男
姓名:lee,性别:女,成绩:90
```
解释:创建了一个Person对象s0,姓名为“wang”,性别为“m”,输出字符串“姓名:wang,性别:男”。创建了一个Student对象s1,姓名为“lee”,性别为“W”,成绩为90,输出字符串“姓名:lee,性别:女,成绩:90”。
用c++语言编写设计人员类Person和日期类date,人员类派生出学生类Student和教师类Teacher。教师类包含姓名、生日、ID、专业、所属机构,学生类包含姓名、ID、成绩、生日,教师类与学生类的数据成员birthday(生日)
可以参考如下代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 日期类
class Date {
public:
Date(int year, int month, int day) : year_(year), month_(month), day_(day) {}
int year() const { return year_; }
int month() const { return month_; }
int day() const { return day_; }
private:
int year_;
int month_;
int day_;
};
// 人员类
class Person {
public:
Person(const string& name, const Date& birthday) : name_(name), birthday_(birthday) {}
string name() const { return name_; }
Date birthday() const { return birthday_; }
private:
string name_;
Date birthday_;
};
// 学生类
class Student : public Person {
public:
Student(const string& name, int id, int score, const Date& birthday)
: Person(name, birthday), id_(id), score_(score) {}
int id() const { return id_; }
int score() const { return score_; }
private:
int id_;
int score_;
};
// 教师类
class Teacher : public Person {
public:
Teacher(const string& name, const Date& birthday, int id, const string& major, const string& institution)
: Person(name, birthday), id_(id), major_(major), institution_(institution) {}
int id() const { return id_; }
string major() const { return major_; }
string institution() const { return institution_; }
private:
int id_;
string major_;
string institution_;
};
int main() {
Date d(1999, 1, 1);
Student s("张三", 1, 90, d);
Teacher t("李四", d, 1001, "计算机科学", "清华大学");
cout << s.name() << " " << s.id() << " " << s.score() << " " << s.birthday().year() << "-" << s.birthday().month() << "-" << s.birthday().day() << endl;
cout << t.name() << " " << t.id() << " " << t.major() << " " << t.institution() << " " << t.birthday().year() << "-" << t.birthday().month() << "-" << t.birthday().day() << endl;
return 0;
}
```
其中,日期类 `Date` 包含年、月、日三个私有成员变量和一个构造函数,以及三个公有成员函数分别返回年、月、日。
人员类 `Person` 包含姓名和生日两个私有成员变量和一个构造函数,以及两个公有成员函数分别返回姓名和生日。
学生类 `Student` 是人员类的派生类,包含ID、成绩两个私有成员变量和一个构造函数,以及两个公有成员函数分别返回ID和成绩。
教师类 `Teacher` 也是人员类的派生类,包含ID、专业、所属机构三个私有成员变量和一个构造函数,以及三个公有成员函数分别返回ID、专业和所属机构。注意,教师类的构造函数需要调用父类 `Person` 的构造函数初始化姓名和生日。同时,由于学生类和教师类都有生日这个数据成员,所以在 `Person` 类中需要提供一个公有的成员函数返回生日。
在 `main` 函数中,创建一个日期对象 `d`,分别创建一个学生对象 `s` 和一个教师对象 `t`,并调用它们的成员函数输出各自的信息。
相关推荐
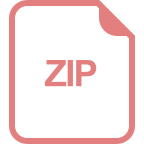
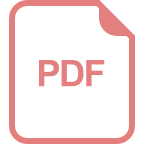











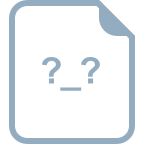
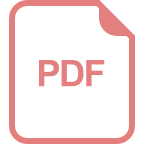