import cv2# 创建一个黑色图像img = np.zeros((200, 400, 3), dtype=np.uint8)# 定义四个数字numbers = [0.08064, 0.96458709, 0.992446, 0.92077314]# 在图像上添加数字for i, num in enumerate(numbers): # 将数字转换为字符串 num_str = str(num) # 设置字体、大小、颜色、粗细等参数 font = cv2.FONT_HERSHEY_SIMPLEX font_scale = 0.7 color = (255, 255, 255) thickness = 2 # 计算文字位置 x = int((i + 0.5) * img.shape[1] / len(numbers)) y = int(img.shape[0] / 2) # 添加文字 cv2.putText(img, num_str, (x, y), font, font_scale, color, thickness)# 显示图像cv2.imshow('Image', img)cv2.waitKey(0)cv2.destroyAllWindows()怎么在显示的数字前添加名称
时间: 2023-09-16 22:11:00 浏览: 64
你可以在添加数字之前使用类似于以下代码的方式添加名称:
```
names = ['Number1', 'Number2', 'Number3', 'Number4'] # 定义名称列表
for i, (num, name) in enumerate(zip(numbers, names)): # 遍历数字和名称列表
# 将数字转换为字符串
num_str = str(num)
# 设置字体、大小、颜色、粗细等参数
font = cv2.FONT_HERSHEY_SIMPLEX
font_scale = 0.7
color = (255, 255, 255)
thickness = 2
# 计算文字位置
x = int((i + 0.5) * img.shape[1] / len(numbers))
y = int(img.shape[0] / 2)
# 添加名称
cv2.putText(img, name, (x, y - 30), font, font_scale, color, thickness)
# 添加数字
cv2.putText(img, num_str, (x, y), font, font_scale, color, thickness)
```
这将在每个数字的上方添加它们的名称。
相关问题
import cv2# 创建一个黑色图像img = np.zeros((200, 400, 3), dtype=np.uint8)# 定义四个数字numbers = [0.08064, 0.96458709, 0.992446, 0.92077314]# 在图像上添加数字for i, num in enumerate(numbers): # 将数字转换为字符串 num_str = str(num) # 设置字体、大小、颜色、粗细等参数 font = cv2.FONT_HERSHEY_SIMPLEX font_scale = 0.7 color = (255, 255, 255) thickness = 2 # 计算文字位置 x = int((i + 0.5) * img.shape[1] / len(numbers)) y = int(img.shape[0] / 2) # 添加文字 cv2.putText(img, num_str, (x, y), font, font_scale, color, thickness)# 显示图像cv2.imshow('Image', img)cv2.waitKey(0)cv2.destroyAllWindows()怎么改数字位置
要改变数字的位置,你需要修改计算文字位置的代码。在这个例子中,x 和 y 的值分别是数字在图像中的横坐标和纵坐标,它们的计算方式如下:
```
x = int((i + 0.5) * img.shape[1] / len(numbers))
y = int(img.shape[0] / 2)
```
其中 `i` 是数字在列表 `numbers` 中的索引,`img.shape[1]` 是图像的宽度,`len(numbers)` 是数字的个数,所以 `int((i + 0.5) * img.shape[1] / len(numbers))` 就是数字在图像中的横坐标。`img.shape[0]` 是图像的高度,所以 `int(img.shape[0] / 2)` 就是数字在图像中的纵坐标。
如果你想要将数字的位置向上或向下移动一些像素,可以调整纵坐标的值。例如,如果你想要将数字向上移动 50 个像素,可以将计算纵坐标的代码修改为:
```
y = int(img.shape[0] / 2) - 50
```
如果你想要将数字向下移动 50 个像素,可以将计算纵坐标的代码修改为:
```
y = int(img.shape[0] / 2) + 50
```
如果你想要将数字的位置向左或向右移动一些像素,可以调整横坐标的值。例如,如果你想要将数字向左移动 50 个像素,可以将计算横坐标的代码修改为:
```
x = int((i + 0.5) * img.shape[1] / len(numbers)) - 50
```
如果你想要将数字向右移动 50 个像素,可以将计算横坐标的代码修改为:
```
x = int((i + 0.5) * img.shape[1] / len(numbers)) + 50
```
你可以根据自己的需要修改这些代码,来达到你想要的数字位置。
import cv2import numpy as np# 读取视频cap = cv2.VideoCapture('video.mp4')# 创建空白图像ret, frame = cap.read()height, width, _ = frame.shapeimg = np.zeros((height, width * 100, 3), dtype=np.uint8)# 逐帧读取视频并将其拼接到图像中i = 0while(cap.isOpened()): ret, frame = cap.read() if ret == False: break img[:, i*width:(i+1)*width, :] = frame i += 1# 保存图像cv2.imwrite('result.jpg', img)# 释放资源cap.release()如何解决ValueError: could not broadcast input array from shape (544,960,3) into shape (544,0,3)
这个错误是因为你创建的空白图像中,第二个维度的大小为width * 100,而在拼接图像时,你的代码没有考虑到这一点。
你需要在拼接图像之前计算好图像的总宽度,然后将空白图像的第二个维度调整为该总宽度。可以使用以下代码来实现:
```
# 读取视频
cap = cv2.VideoCapture('video.mp4')
# 创建空白图像
ret, frame = cap.read()
height, width, _ = frame.shape
total_width = width * 100 # 计算图像的总宽度
img = np.zeros((height, total_width, 3), dtype=np.uint8)
# 逐帧读取视频并将其拼接到图像中
i = 0
while(cap.isOpened()):
ret, frame = cap.read()
if ret == False:
break
img[:, i*width:(i+1)*width, :] = frame
i += 1
# 保存图像
cv2.imwrite('result.jpg', img)
# 释放资源
cap.release()
```
在这个修改后的代码中,total_width变量用于计算图像的总宽度,img数组的第二个维度被调整为total_width,以便在拼接图像时不会出现维度不匹配的错误。
相关推荐
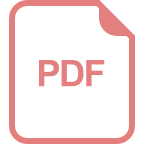
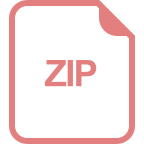














