C编程(1)当数据类型不是整型,而是如下结构体类型: Typedef struct student { char name[8]; int score; } Student a[n]; 修改源程序,通过从键盘输入n个学生的考试成绩表,按分数高低次序,打印出每个学生的信息。
时间: 2023-11-27 07:52:12 浏览: 44
下面是一个示例代码:
```c
#include <stdio.h>
#include <string.h>
typedef struct student {
char name[8];
int score;
} Student;
int main() {
int n, i, j;
printf("请输入学生人数:");
scanf("%d", &n);
Student a[n];
// 从键盘输入学生信息
for (i = 0; i < n; i++) {
printf("请输入第%d个学生的姓名和分数:", i+1);
scanf("%s%d", a[i].name, &a[i].score);
}
// 按分数从高到低排序
for (i = 0; i < n-1; i++) {
for (j = 0; j < n-i-1; j++) {
if (a[j].score < a[j+1].score) {
Student temp = a[j];
a[j] = a[j+1];
a[j+1] = temp;
}
}
}
// 打印学生信息
printf("按分数从高到低排序的学生信息如下:\n");
for (i = 0; i < n; i++) {
printf("姓名:%s\t分数:%d\n", a[i].name, a[i].score);
}
return 0;
}
```
此代码中,我们首先定义了一个名为 `Student` 的结构体类型,它包含两个成员,一个是 `name`,表示学生的姓名,类型为字符数组,长度为8;另一个是 `score`,表示学生的分数,类型为整型。
接着,在 `main` 函数中,我们首先从键盘输入学生人数 `n`,并声明一个 `Student` 类型的数组 `a`,长度为 `n`。
然后,我们使用循环从键盘输入每个学生的信息,并将其存储到数组 `a` 中。
接下来,我们使用冒泡排序算法按照学生的分数从高到低对学生信息进行排序。
最后,我们使用循环打印排序后的学生信息。
相关推荐
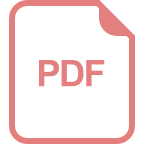
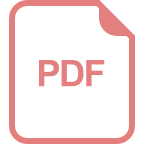
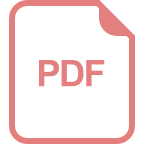
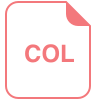
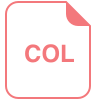
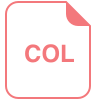
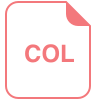










