详细地教我用Qt制作一个函数图像绘制器,要求能够输入任意函数,点击设置好的ok键后,把输入的函数转化成函数图像并在坐标轴中呈现出来,,生成的函数图像可以叠加,有一个清除键可以清楚之前生成的所有函数图像,有一个保存键可以保存当前的函数图像并且可以选择保存地址,转化为jpg图片文件
时间: 2023-11-27 12:55:38 浏览: 95
好的,我们可以分为以下几个步骤来实现:
1. 创建GUI界面:在Qt Creator中新建一个工程,选择Qt Widgets Application类型,创建一个主窗口,添加需要的控件,包括一个文本框用于输入函数,一个按钮用于确认输入,一个按钮用于清除画布,一个按钮用于保存画布,一个QCustomPlot控件用于绘制函数图像。
2. 实现函数解析:通过调用QString的toStdString()函数将输入的函数转换成std::string类型,再使用数学表达式解析库来计算函数值。我们推荐使用muParserX库,可以在Qt工程中直接使用。
3. 绘制函数图像:定义一个函数,接收一个函数字符串,以及绘制参数(例如颜色、线宽等),然后通过遍历x轴坐标,计算出对应的y轴坐标,使用QCustomPlot控件绘制出函数图像。
4. 清除画布:定义一个函数,遍历所有已绘制的函数图像,删除它们,并重新绘制一个空白的坐标轴。
5. 保存画布:定义一个函数,调用QCustomPlot控件的saveJpg()函数,将当前绘制的图像保存为jpg格式。
下面是实现代码的示例,你可以在此基础上进行修改和完善:
mainwindow.h:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "muparserx/muparserx.h"
#include "qcustomplot/qcustomplot.h"
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_confirmButton_clicked();
void on_clearButton_clicked();
void on_saveButton_clicked();
private:
Ui::MainWindow *ui;
mu::ParserX parser;
QVector<QCPGraph*> graphs;
QColor colors[5] = {Qt::red, Qt::green, Qt::blue, Qt::cyan, Qt::magenta};
int colorIndex = 0;
void drawFunction(QString function, QColor color, double lineWidth);
void clearGraphs();
void saveGraph();
};
#endif // MAINWINDOW_H
```
mainwindow.cpp:
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QMessageBox>
#include <QFileDialog>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 设置坐标轴范围和名称
ui->customPlot->xAxis->setRange(-10, 10);
ui->customPlot->yAxis->setRange(-10, 10);
ui->customPlot->xAxis->setLabel("x");
ui->customPlot->yAxis->setLabel("y");
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_confirmButton_clicked()
{
QString function = ui->functionEdit->text();
if(function.isEmpty()) {
QMessageBox::warning(this, "Error", "Please input a function!");
return;
}
// 设置解析器变量和函数
parser.DefineVar("x", &mu::ParserX::Variable);
parser.SetExpr(function.toStdString());
// 绘制函数图像
drawFunction(function, colors[colorIndex++ % 5], 2);
}
void MainWindow::on_clearButton_clicked()
{
clearGraphs();
}
void MainWindow::on_saveButton_clicked()
{
QString filePath = QFileDialog::getSaveFileName(this, tr("Save Image"), "", tr("JPEG (*.jpg)"));
if(!filePath.isEmpty()) {
saveGraph();
ui->customPlot->saveJpg(filePath);
}
}
void MainWindow::drawFunction(QString function, QColor color, double lineWidth)
{
QVector<double> xData, yData;
double xMin = ui->customPlot->xAxis->range().lower;
double xMax = ui->customPlot->xAxis->range().upper;
double xStep = (xMax - xMin) / 100;
for(double x = xMin; x <= xMax; x += xStep) {
parser.SetVar("x", x);
double y = parser.Eval();
xData.append(x);
yData.append(y);
}
QCPGraph *graph = ui->customPlot->addGraph();
graph->setData(xData, yData);
graph->setPen(QPen(color, lineWidth));
graphs.append(graph);
ui->customPlot->replot();
}
void MainWindow::clearGraphs()
{
for(auto graph : graphs) {
ui->customPlot->removeGraph(graph);
}
graphs.clear();
ui->customPlot->replot();
}
void MainWindow::saveGraph()
{
ui->customPlot->toPixmap().save("temp.jpg");
}
```
在这个示例中,我们使用了muParserX库来进行函数解析,使用QCustomPlot控件来进行函数图像绘制。其中,drawFunction()函数用于绘制函数图像,clearGraphs()函数用于清除画布,saveGraph()函数用于保存画布,每个函数都有相应的按钮与之对应,可以在mainwindow.ui中设置。你需要自己安装muParserX库和QCustomPlot控件,这些库可以在Qt Creator中直接安装。
阅读全文
相关推荐
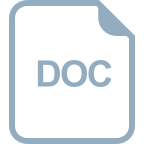
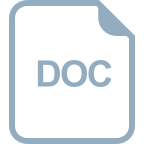
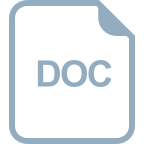






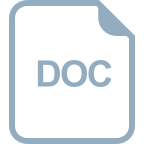
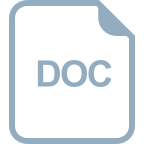
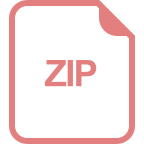
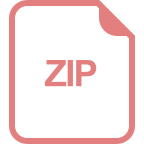
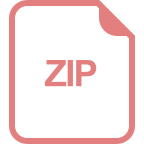
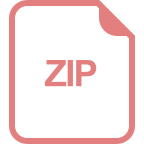
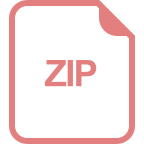