一个系统中有几千个ip,每5分钟更新一次ip的在线状态,并存入数据库,服务部署在linux机器上,可以通过ping来拿到ip的在离线信息,如何高效完成。可使用多线程,给出一个完美、高效的方案,并给出java代码
时间: 2023-12-07 09:03:07 浏览: 78
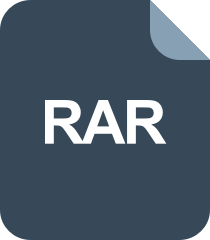
编程实现检测一个ip是否在线的ping操作
针对这个需求,可以使用多线程来并发地进行ping操作,以提高效率。具体的方案如下:
1. 将IP地址分为多个子集,每个子集中的IP数量适当,可以根据服务器性能和网络情况来调整。
2. 开启多个线程,每个线程负责一个IP子集的ping操作,通过Runtime.getRuntime().exec()方法来执行ping命令,例如:
```java
String ipAddress = "192.168.0.1";
Process process = Runtime.getRuntime().exec("ping -c 1 -w 100 " + ipAddress);
int result = process.waitFor();
if (result == 0) {
// 在线
} else {
// 离线
}
```
3. 将每个IP的在线状态存入数据库中,可以使用连接池来提高效率。
4. 使用定时任务来定时执行线程,每次执行完后等待一段时间再次执行,例如每5分钟执行一次。
下面是完整的Java代码示例:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class IPStatusChecker {
// IP地址列表
private static final List<String> ipList = new ArrayList<>();
public static void main(String[] args) {
// 初始化IP地址列表
for (int i = 1; i <= 1000; i++) {
ipList.add("192.168.0." + i);
}
// 创建线程池
int threadCount = 10;
ExecutorService executorService = Executors.newFixedThreadPool(threadCount);
// 每个线程负责一个子集的IP地址
int ipCountPerThread = ipList.size() / threadCount;
for (int i = 0; i < threadCount; i++) {
int startIndex = i * ipCountPerThread;
int endIndex = (i + 1) * ipCountPerThread;
if (i == threadCount - 1) {
endIndex = ipList.size();
}
List<String> subList = ipList.subList(startIndex, endIndex);
// 创建线程
executorService.execute(new IPStatusCheckerThread(subList));
}
// 关闭线程池
executorService.shutdown();
}
// 检查IP地址在线状态的线程
private static class IPStatusCheckerThread implements Runnable {
private final List<String> subList;
public IPStatusCheckerThread(List<String> subList) {
this.subList = subList;
}
@Override
public void run() {
try {
for (String ipAddress : subList) {
// 执行ping命令
Process process = Runtime.getRuntime().exec("ping -c 1 -w 100 " + ipAddress);
int result = process.waitFor();
if (result == 0) {
// 在线
System.out.println(ipAddress + " is online");
} else {
// 离线
System.out.println(ipAddress + " is offline");
}
// 将在线状态存入数据库中
// ...
// 等待一段时间再次执行
Thread.sleep(100);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
```
需要注意的是,本方案仅适用于内网环境下的IP地址在线状态检测,因为在公网环境下,很多IP地址是不允许ping的。如果需要在公网环境下进行IP地址在线状态检测,可以考虑使用其他方式,例如TCP连接或HTTP请求。
阅读全文
相关推荐
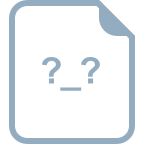
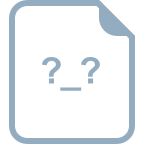
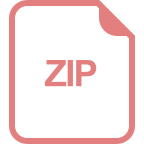
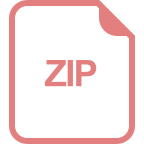
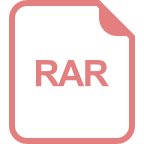
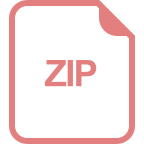
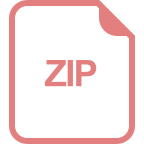
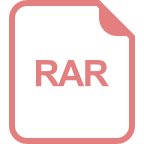
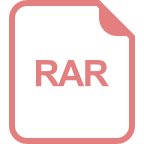
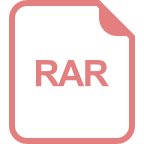
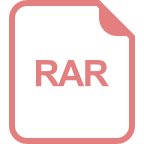
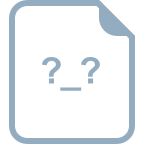
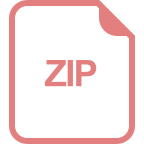
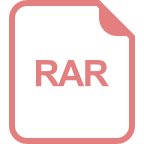
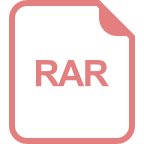
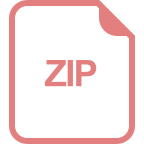