编写一款图书管理系统的Java代码
时间: 2024-03-24 09:37:11 浏览: 22
好的,以下是一份简单的图书管理系统的Java代码:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class BookManagementSystem {
private ArrayList<Book> bookList;
public BookManagementSystem() {
bookList = new ArrayList<Book>();
}
public void addBook(Book book) {
bookList.add(book);
}
public void removeBook(Book book) {
bookList.remove(book);
}
public void displayBooks() {
if (bookList.size() == 0) {
System.out.println("There are no books in the library.");
} else {
for (Book book : bookList) {
System.out.println(book);
}
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
BookManagementSystem system = new BookManagementSystem();
while (true) {
System.out.println("1. Add book");
System.out.println("2. Remove book");
System.out.println("3. Display books");
System.out.println("4. Exit");
System.out.print("Enter your choice: ");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.print("Enter book title: ");
String title = scanner.nextLine();
System.out.print("Enter book author: ");
String author = scanner.nextLine();
System.out.print("Enter book ISBN: ");
String isbn = scanner.nextLine();
Book book = new Book(title, author, isbn);
system.addBook(book);
break;
case 2:
System.out.print("Enter book title: ");
title = scanner.nextLine();
System.out.print("Enter book author: ");
author = scanner.nextLine();
System.out.print("Enter book ISBN: ");
isbn = scanner.nextLine();
book = new Book(title, author, isbn);
system.removeBook(book);
break;
case 3:
system.displayBooks();
break;
case 4:
System.exit(0);
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
}
class Book {
private String title;
private String author;
private String isbn;
public Book(String title, String author, String isbn) {
this.title = title;
this.author = author;
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getIsbn() {
return isbn;
}
@Override
public String toString() {
return "Title: " + title + ", Author: " + author + ", ISBN: " + isbn;
}
}
```
这份代码实现了以下功能:
1. 添加图书
2. 删除图书
3. 显示所有图书
4. 退出程序
每本图书由书名、作者和ISBN号组成。用户可以根据自己的需求添加、删除和查看图书。
相关推荐
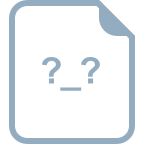
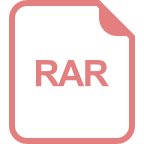
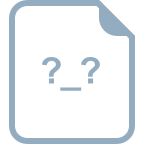














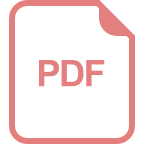