51单片机使用compim进行通信,不连接P3.0、P3.1引脚,不用SBUF,而是将收发接到其他引脚,用定时器和外部中断,写个一串口收发程序,具备波特率设置功能、具备发送16进制数据功能、printf可调用。
时间: 2024-09-10 16:27:24 浏览: 140
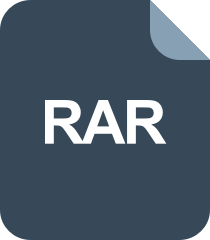
如何在51单片机上实现串口收发命令-综合文档
在51单片机上使用COMPIII进行通信,而不用P3.0和P3.1引脚,可以通过软件模拟串口的方式来实现。不过,这需要精细的定时器控制来模拟串口的波特率,以及使用外部中断来检测起始位和处理数据位。由于这个过程比较复杂,通常会依赖于硬件的特性来简化编程。下面是一个简化的例子,展示如何实现这一过程:
首先,你需要设置定时器来产生正确的波特率。波特率的计算依赖于晶振频率,这里假设使用的是11.0592MHz的晶振。
```c
#include <reg51.h>
#define FOSC 11059200L // Oscillator frequency
#define BAUD 9600 // UART baudrate
void Timer0_Init() {
TMOD &= 0xF0; // Clear timer 0 mode bits
TMOD |= 0x01; // Timer 0 mode 1
TH0 = (65536 - (FOSC/12/32)/BAUD)/256;
TL0 = (65536 - (FOSC/12/32)/BAUD)%256;
ET0 = 1; // Enable Timer 0 interrupt
EA = 1; // Enable global interrupt
TR0 = 1; // Start Timer 0
}
void SendByte(unsigned char byte) {
SBUF = byte; // Place the data in SBUF for transmission
while (!TI); // Wait for the transmission to complete
TI = 0; // Clear the transmission interrupt flag
}
void SerialSend(unsigned char *str) {
while (*str) {
SendByte(*str++);
}
}
void main() {
Timer0_Init(); // Initialize Timer 0
while (1) {
SerialSend("Hello, COMPIII!\r\n"); // Send some data
}
}
void Timer0_ISR (void) interrupt 1 {
static unsigned char bitCount = 0;
static unsigned char sreg;
TH0 = (65536 - (FOSC/12/32)/BAUD)/256;
TL0 = (65536 - (FOSC/12/32)/BAUD)%256;
if (bitCount == 0) {
if (RI) { // If receive interrupt flag is set
RI = 0; // Clear the RI flag
sreg = SBUF; // Read the received data
bitCount = 8; // 8 bits of data to be received
}
} else {
bitCount--;
// Process received data, bit by bit
}
}
```
注意,这个例子并没有完全实现软件模拟串口的所有功能,如数据位的逐位处理和printf的重定向。为了完整实现这些功能,你需要根据实际的需求进一步编写代码,可能还需要编写复杂的中断服务程序和数据处理逻辑。
阅读全文
相关推荐
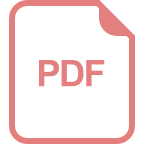
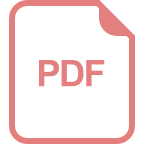



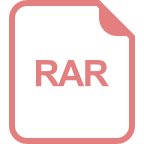
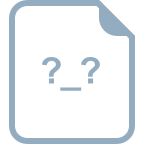
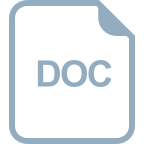
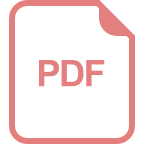
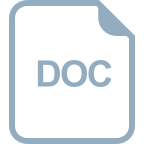
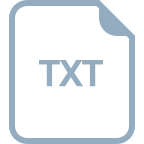
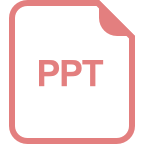
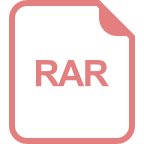
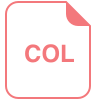
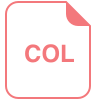
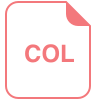
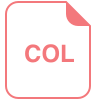
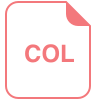