c语言用二叉排序树通讯录管理系统
时间: 2023-09-27 13:08:28 浏览: 151
二叉排序树通讯录管理系统可以通过C语言实现,下面是一个简单的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
#define MAX_TEL_LEN 11
// 通讯录结构体
typedef struct _contact {
char name[MAX_NAME_LEN]; // 姓名
char tel[MAX_TEL_LEN]; // 电话
struct _contact *lchild; // 左子节点
struct _contact *rchild; // 右子节点
} Contact;
// 创建通讯录节点
Contact *create_contact(char *name, char *tel) {
Contact *contact = (Contact *)malloc(sizeof(Contact));
if (contact == NULL) {
fprintf(stderr, "Failed to allocate memory for contact!\n");
exit(1);
}
strncpy(contact->name, name, MAX_NAME_LEN);
strncpy(contact->tel, tel, MAX_TEL_LEN);
contact->lchild = NULL;
contact->rchild = NULL;
return contact;
}
// 插入通讯录节点
void insert_contact(Contact **root, Contact *contact) {
if (*root == NULL) {
*root = contact;
} else if (strcmp((*root)->name, contact->name) >= 0) {
insert_contact(&(*root)->lchild, contact);
} else {
insert_contact(&(*root)->rchild, contact);
}
}
// 查找通讯录节点
Contact *search_contact(Contact *root, char *name) {
if (root == NULL) {
return NULL;
}
if (strcmp(root->name, name) == 0) {
return root;
} else if (strcmp(root->name, name) > 0) {
return search_contact(root->lchild, name);
} else {
return search_contact(root->rchild, name);
}
}
// 删除通讯录节点
void delete_contact(Contact **root, char *name) {
Contact *p, *q, *s;
if (*root == NULL) {
return;
}
p = *root;
q = NULL;
while (p != NULL && strcmp(p->name, name) != 0) {
q = p;
if (strcmp(p->name, name) > 0) {
p = p->lchild;
} else {
p = p->rchild;
}
}
if (p == NULL) {
return;
}
if (p->lchild == NULL) {
s = p->rchild;
} else if (p->rchild == NULL) {
s = p->lchild;
} else {
s = p->rchild;
while (s->lchild != NULL) {
s = s->lchild;
}
s->lchild = p->lchild;
s = p->rchild;
}
if (q == NULL) {
*root = s;
} else if (q->lchild == p) {
q->lchild = s;
} else {
q->rchild = s;
}
free(p);
}
// 打印通讯录
void print_contact(Contact *root) {
if (root == NULL) {
return;
}
print_contact(root->lchild);
printf("%s\t%s\n", root->name, root->tel);
print_contact(root->rchild);
}
int main() {
Contact *root = NULL;
char name[MAX_NAME_LEN];
char tel[MAX_TEL_LEN];
int opt;
printf("Welcome to Contact Management System!\n");
while (1) {
printf("\n1. Insert Contact\n");
printf("2. Search Contact\n");
printf("3. Delete Contact\n");
printf("4. Print Contact List\n");
printf("5. Exit\n");
printf("Please select an option: ");
scanf("%d", &opt);
switch (opt) {
case 1:
printf("Please enter name: ");
scanf("%s", name);
printf("Please enter telephone: ");
scanf("%s", tel);
insert_contact(&root, create_contact(name, tel));
printf("Contact inserted successfully!\n");
break;
case 2:
printf("Please enter name: ");
scanf("%s", name);
Contact *contact = search_contact(root, name);
if (contact != NULL) {
printf("Name: %s\tTelephone: %s\n", contact->name, contact->tel);
} else {
printf("Contact not found!\n");
}
break;
case 3:
printf("Please enter name: ");
scanf("%s", name);
delete_contact(&root, name);
printf("Contact deleted successfully!\n");
break;
case 4:
printf("Name\tTelephone\n");
print_contact(root);
break;
case 5:
printf("Goodbye!\n");
exit(0);
default:
printf("Invalid option!\n");
}
}
return 0;
}
```
这个程序实现了一个简单的通讯录管理系统,使用二叉排序树存储通讯录信息,支持插入、查找、删除和打印通讯录等操作。
阅读全文
相关推荐
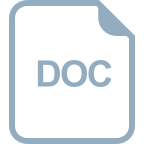
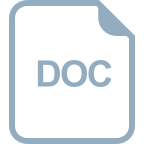
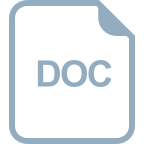

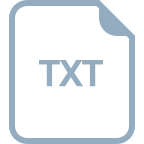
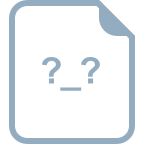
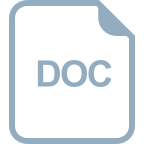
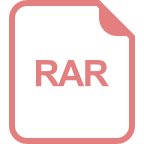
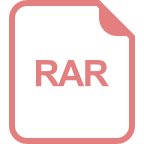
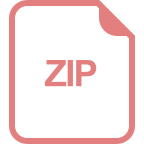
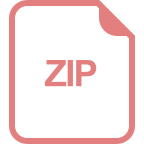
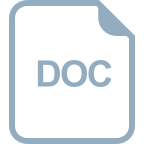
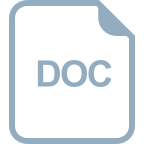
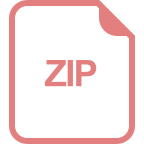
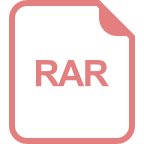
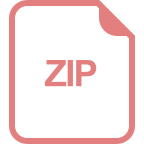
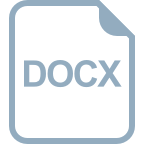
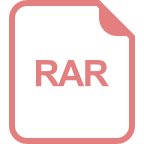
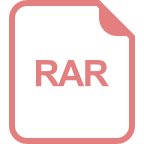