c语言 随机输入四个字符串st1[10]、st2[10]、st3[10]、st4[10],输出它们中最大字符串。
时间: 2023-11-24 18:05:34 浏览: 137
您好,我已经明白了您的要求。下面是 C 语言代码实现:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char st1[10], st2[10], st3[10], st4[10], max[10];
printf("请输入四个字符串:\n");
scanf("%s %s %s %s", st1, st2, st3, st4);
strcpy(max, st1); // 假设第一个字符串最大
if (strcmp(max, st2) < 0)
{
strcpy(max, st2);
}
if (strcmp(max, st3) < 0)
{
strcpy(max, st3);
}
if (strcmp(max, st4) < 0)
{
strcpy(max, st4);
}
printf("最大的字符串是:%s\n", max);
return 0;
}
```
在这个程序中,我们使用了 `scanf()` 函数输入四个字符串,并且使用 `strcpy()` 函数将假设的最大字符串复制到变量 `max` 中。然后依次使用 `strcmp()` 函数与其余三个字符串进行比较,如果发现更大的字符串就将其复制到 `max` 中。最后输出 `max` 就是最大的字符串了。
相关问题
C语言随机输入四个字符串st1[10]、st2[10]、st3[10]、st4[10],输出它们中最大字符串。
可以使用strcmp()函数来比较四个字符串的大小关系,依次比较st1,st2,st3和st4的大小,最终得到最大字符串。代码如下:
#include <stdio.h>
#include <string.h> /* for strcmp() */
int main()
{
char st1[10], st2[10], st3[10], st4[10]; /* 声明四个字符串变量 */
char max[10]; /* 声明用于存储最大字符串的变量 */
printf("请输入四个字符串:");
scanf("%s %s %s %s", st1, st2, st3, st4);
/* 依次比较四个字符串,并存储最大字符串 */
strcpy(max, st1);
if (strcmp(st2, max) > 0) strcpy(max, st2);
if (strcmp(st3, max) > 0) strcpy(max, st3);
if (strcmp(st4, max) > 0) strcpy(max, st4);
printf("最大的字符串是:%s\n", max);
return 0;
}
c语言 随机输入四个字符串st1[10]、st2[10]、st3[10]、st4[10],输出它们中最大的字符。
好的,让我来回答你的问题。以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char st1[10], st2[10], st3[10], st4[10];
char max = '\0';
printf("请输入四个字符串:\n");
scanf("%s%s%s%s", st1, st2, st3, st4);
for (int i = 0; i < strlen(st1); i++) {
if (st1[i] > max) {
max = st1[i];
}
}
for (int i = 0; i < strlen(st2); i++) {
if (st2[i] > max) {
max = st2[i];
}
}
for (int i = 0; i < strlen(st3); i++) {
if (st3[i] > max) {
max = st3[i];
}
}
for (int i = 0; i < strlen(st4); i++) {
if (st4[i] > max) {
max = st4[i];
}
}
printf("最大的字符是:%c\n", max);
return 0;
}
```
该代码使用了四个scanf()函数从标准输入中读入四个字符串,然后使用了一个for循环逐个比较每个字符串的每个字符,找出最大的字符并将其输出。
希望这个回答能对你有所帮助。
阅读全文
相关推荐
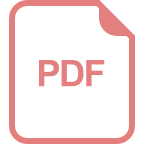
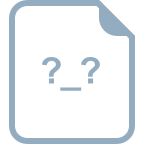



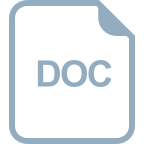








