.假设有一张成绩表记录了10名学生的语文、数学、英语、生物、历史这5门课的成绩,成绩范围均为50~100分。10名学生的学号分别为100、101、102、103、104、105、106、107、108、109。利用numpy完成一下操作: (1)使用随机数模拟学生成绩,并存储在数组中。 (2)查询学号为105的学生的英语成绩。 (3)查询学号为100、102、105、109的4位学生的语文、数学和英语成绩。 (4)查询大于或等于90分的成绩和相应学生的学号。 (5)按各门课程的成绩排序。 (6)按每名学生的成绩排序。 (7)计算每门课程的平均分、最高分和最低分。 (8)计算每名学生的最高分、最低分。 (9)查询最低分及相应的学生学号和课程。 (10)查询最高分及相应的学生学号和课程。 (11)语文、数学、英语、生物、历史这5门课程在总分中的占比分别为25%、25%、20%、15%、15%。如果总分位100分,则计算每名学生的总成绩。 (12)查询最高的3个总分。
时间: 2023-12-16 18:22:52 浏览: 63
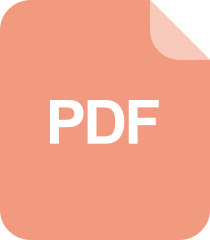
学科、姓名、分数。 分别计算每门学科分数最高的前三名,并显示名次。写入MySQL中。
好的,我来回答你的问题:
(1) 代码实现:
```python
import numpy as np
# 使用随机数模拟学生成绩,并存储在数组中
scores = np.random.randint(50, 101, size=(10, 5))
ids = np.array([100, 101, 102, 103, 104, 105, 106, 107, 108, 109])
print('学生成绩:')
print(scores)
```
(2) 代码实现:
```python
# 查询学号为105的学生的英语成绩
en_score_105 = scores[np.where(ids == 105), 2]
print('学号为105的学生的英语成绩:')
print(en_score_105)
```
(3) 代码实现:
```python
# 查询学号为100、102、105、109的4位学生的语文、数学和英语成绩
selected_ids = np.array([100, 102, 105, 109])
selected_scores = scores[np.isin(ids, selected_ids), :3]
print('4位学生的语文、数学和英语成绩:')
print(selected_scores)
```
(4) 代码实现:
```python
# 查询大于或等于90分的成绩和相应学生的学号
high_scores = scores[np.where(scores >= 90)]
high_ids = ids[np.where(scores >= 90)]
print('大于或等于90分的成绩和相应学生的学号:')
for i in range(len(high_scores)):
print('学号:', high_ids[i], ' 成绩:', high_scores[i])
```
(5) 代码实现:
```python
# 按各门课程的成绩排序
sorted_scores = np.sort(scores, axis=0)
print('按各门课程的成绩排序:')
print(sorted_scores)
```
(6) 代码实现:
```python
# 按每名学生的成绩排序
sorted_scores = np.sort(scores, axis=1)
print('按每名学生的成绩排序:')
print(sorted_scores)
```
(7) 代码实现:
```python
# 计算每门课程的平均分、最高分和最低分
mean_scores = np.mean(scores, axis=0)
max_scores = np.max(scores, axis=0)
min_scores = np.min(scores, axis=0)
print('每门课程的平均分、最高分和最低分:')
for i in range(len(mean_scores)):
print('科目:', i, ' 平均分:', mean_scores[i], ' 最高分:', max_scores[i], ' 最低分:', min_scores[i])
```
(8) 代码实现:
```python
# 计算每名学生的最高分、最低分
max_scores = np.max(scores, axis=1)
min_scores = np.min(scores, axis=1)
print('每名学生的最高分、最低分:')
for i in range(len(max_scores)):
print('学号:', ids[i], ' 最高分:', max_scores[i], ' 最低分:', min_scores[i])
```
(9) 代码实现:
```python
# 查询最低分及相应的学生学号和课程
min_score = np.min(scores)
min_index = np.where(scores == min_score)
min_student_id = ids[min_index[0][0]]
min_course = min_index[1][0]
print('最低分及相应的学生学号和课程:')
print('学号:', min_student_id, ' 课程:', min_course, ' 最低分:', min_score)
```
(10) 代码实现:
```python
# 查询最高分及相应的学生学号和课程
max_score = np.max(scores)
max_index = np.where(scores == max_score)
max_student_id = ids[max_index[0][0]]
max_course = max_index[1][0]
print('最高分及相应的学生学号和课程:')
print('学号:', max_student_id, ' 课程:', max_course, ' 最高分:', max_score)
```
(11) 代码实现:
```python
# 计算每名学生的总成绩
weights = np.array([0.25, 0.25, 0.2, 0.15, 0.15])
total_scores = np.dot(scores, weights) # 矩阵乘法
print('每名学生的总成绩:')
for i in range(len(total_scores)):
print('学号:', ids[i], ' 总成绩:', total_scores[i])
```
(12) 代码实现:
```python
# 查询最高的3个总分
top_k = 3
sorted_indices = np.argsort(total_scores)[::-1][:top_k]
sorted_scores = total_scores[sorted_indices]
sorted_ids = ids[sorted_indices]
print('最高的3个总分:')
for i in range(len(sorted_scores)):
print('排名:', i+1, ' 学号:', sorted_ids[i], ' 总成绩:', sorted_scores[i])
```
阅读全文
相关推荐
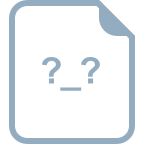









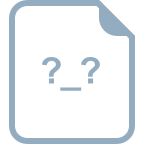
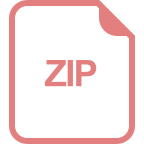
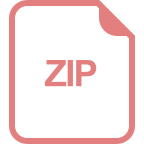
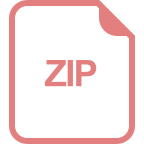
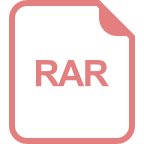
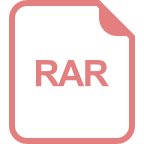