s=input("please input a string:") #********begin******** #********end******** print("the length is:",len) print("the counter is:",counter) 补充程序,程序功能为:输入一段英文,求其字符串长度,并求出其中包含多少个单词。
时间: 2023-07-05 07:36:46 浏览: 99
好的,以下是 Python 代码实现:
```python
s = input("Please input a string:")
# 将字符串按空格分隔,得到单词列表
words = s.split()
# 统计单词个数
counter = len(words)
# 计算字符串长度
length = len(s)
print("The length of the string is:", length)
print("The number of words in the string is:", counter)
```
这段代码会从用户输入中读取一段英文字符串,然后将其按空格分隔成单词列表,统计单词个数和字符串长度,最后输出。其中,字符串长度可以通过 Python 的内置函数 `len()` 来计算,而单词个数则可以通过将字符串按空格分隔成列表,然后对列表的长度进行统计得到。
相关问题
完善下列代码要求如下已知游戏中常见人物状态State抽象类,包括站立(Idle),行走(Run),攻击(Attack),死亡(Dead)等动作。在游戏中有角色hero类,拥有人物状态同时还拥有血量,攻击力,防御力等属性,有怪物类Monster,怪物有血量,攻击力,防御力,怪物类型等属性。 已知角色可以变身为怪物,现在main函数中,模拟某个角色在正常情况下以及在变身状态下的各种状态操作(输入1为正常hero,输入2则变身为monster)。 要求,使用抽象类及的基类指针实现。 #include <iostream> using namespace std; #include<string> #include"time.h" #include"math.h" //请在begin和end之间完成完成代码编写 /*************begin***********/ /*************end**********/ int main() { State *s; /*************begin*****************/ /**************end***************/ s->idle(); s->attack(); s->run(); s->dead(); return 1; }
#include <iostream>
#include <string>
#include <time.h>
#include <math.h>
using namespace std;
// 人物状态抽象类
class State {
public:
virtual void idle() = 0;
virtual void run() = 0;
virtual void attack() = 0;
virtual void dead() = 0;
};
// 人物类
class Hero : public State {
private:
int hp;
int attack;
int defense;
public:
Hero(int h, int a, int d) : hp(h), attack(a), defense(d) {}
virtual void idle() { cout << "Hero is idling." << endl; }
virtual void run() { cout << "Hero is running." << endl; }
virtual void attack() { cout << "Hero is attacking." << endl; }
virtual void dead() { cout << "Hero is dead." << endl; }
};
// 怪物类
class Monster : public State {
private:
int hp;
int attack;
int defense;
string type;
public:
Monster(int h, int a, int d, string t) : hp(h), attack(a), defense(d), type(t) {}
virtual void idle() { cout << "Monster is idling." << endl; }
virtual void run() { cout << "Monster is running." << endl; }
virtual void attack() { cout << "Monster is attacking." << endl; }
virtual void dead() { cout << "Monster is dead." << endl; }
};
int main() {
State *s;
int input;
cout << "Please input 1 for Hero or 2 for Monster: ";
cin >> input;
if (input == 1) {
Hero hero(100, 10, 5);
s = &hero;
}
else if (input == 2) {
Monster monster(80, 15, 3, "Dragon");
s = &monster;
}
else {
cout << "Invalid input." << endl;
return 0;
}
s->idle();
s->attack();
s->run();
s->dead();
return 1;
}
#include <iostream> using namespace std; #define STR_LEN 80 //读入一行存入字符数组str,长度不超过maxLength void readline(char str[], int maxLength); //判断字符串str是否是回文 bool isPalindrome(char str[]); int main() { char str[STR_LEN + 1]; // cout << "Please input a string with length <= " << STR_LEN << ":"; readline(str, STR_LEN); if (isPalindrome(str)) { cout << "Yes" << endl; } else { cout << "No" << endl; } return 0; } //读入一行存入字符数组str,长度不超过maxLength void readline(char str[], int maxLength) { int i = 0; while (i < maxLength) { char ch = cin.get(); if (ch != '\n' && ch != '\r') { str[i] = ch; } else { break; } i++; } str[i] = '\0'; } //判断字符串str是否是回文 bool isPalindrome(char str[]) { /********* Begin *********/ int i=0; while(str[i]!='\0') { i++; } if(str[0]>='A'&&str[0]<='Z') str[0]=str[0]+32; bool flag=true; for(int k=0;k<i/2;k++) { if(str[k]!=str[i-1-k]) { flag=false; break; } } return flag; /********* End *********/ }
这段代码实现了读入一个字符串并判断是否为回文字符串的功能。其中 `readline` 函数实现了从标准输入读取一行字符串的功能,`isPalindrome` 函数实现了判断一个字符串是否为回文字符串的功能。代码中使用了双指针的思想,每次比较字符串的左右端点,如果相同则继续比较,否则说明不是回文字符串。
需要注意的是,该代码只判断了字符串中的英文字母是否为回文,对于数字、符号等字符并未进行处理。如果需要判断多种字符类型,可以在比较字符前进行字符类型的判断和转换。
另外需要注意的是,代码中将大写字母转换为小写字母进行比较,这是因为在判断回文字符串时大小写字母应该是等价的。
阅读全文
相关推荐
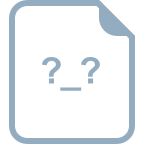
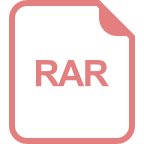
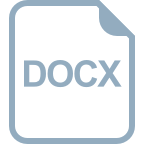
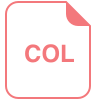
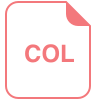
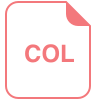









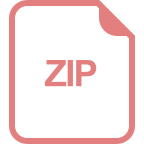