这是我所加的注意力机制模块:class SelfAttention(nn.Module): def init(self, in_channels, reduction=4): super(SelfAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool1d(1) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): # print("x=", x) b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x),然后运行训练程序时报错:File "/root/autodl-tmp/project/tools/../lib/net/pointnet2_msg.py", line 91, in forward y = self.fc1(y) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/module.py", line 727, in _call_impl result = self.forward(*input, **kwargs) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/conv.py", line 258, in forward return F.conv1d(input, self.weight, self.bias, self.stride, RuntimeError: Given groups=1, weight of size [256, 1024, 1], expected input[16, 512, 1] to have 1024 channels, but got 512 channels instead你知道是为什么吗,我该如何解决?请提供详细的解决代码
时间: 2023-11-13 11:03:20 浏览: 19
这个错误的原因是输入的维度不匹配,具体来说,你的输入张量的形状是 `[batch_size, in_channels, n]`,其中 `in_channels` 表示输入特征的通道数,而你的第一个卷积层的输入通道数是 `in_channels // reduction`,即 `in_channels` 除以 `reduction`,这个值应该要与输入张量的 `in_channels` 相等才行。
根据你提供的错误信息,可以看到输入张量的形状是 `[16, 512, 1]`,其中 `in_channels` 是 512,而第一个卷积层的输入通道数应该是 256,这两个值不相等,导致了错误。
为了解决这个问题,你需要在初始化 `SelfAttention` 类时将 `in_channels` 设置为正确的值。具体来说,你需要在使用 `SelfAttention` 的地方,将其初始化时的 `in_channels` 设置为输入张量的 `in_channels`,例如:
```
# 假设你的输入张量的形状是 [batch_size, in_channels, n]
sa = SelfAttention(in_channels=in_channels, reduction=4)
y = sa(x)
```
在这里,我们将输入张量的 `in_channels` 传递给 `SelfAttention` 的初始化函数,构建注意力机制模块时使用该值。
另外,你的 `SelfAttention` 类的 `__init__` 方法中,应该将 `super(SelfAttention, self).__init__()` 改为 `super(SelfAttention, self).__init__()`,这是一个小错误。
最终的代码应该如下所示:
```
class SelfAttention(nn.Module):
def __init__(self, in_channels, reduction=4):
super(SelfAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool1d(1)
self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False)
self.relu = nn.ReLU(inplace=True)
self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
b, c, n = x.size()
y = self.avg_pool(x)
y = self.fc1(y)
y = self.relu(y)
y = self.fc2(y)
y = self.sigmoid(y)
return x * y.expand_as(x)
```
希望能对你有所帮助!
相关推荐
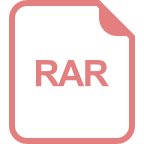
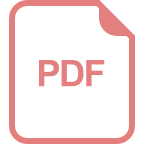
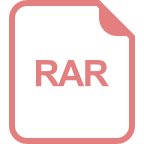








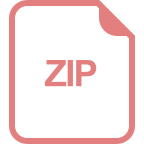
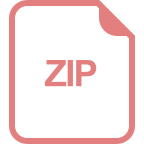
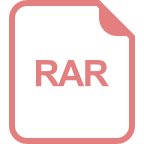
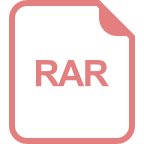
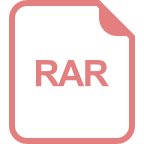
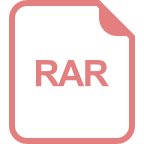