pycharm写游戏需要怎么安装模块
时间: 2023-12-24 12:03:02 浏览: 140
为了在PyCharm中编写游戏,您需要安装一些游戏库和模块。具体来说,您需要通过以下命令安装pygame模块:
pip install pygame
除了pygame模块外,对于特定类型的游戏,您可能需要安装其他模块,例如pyopengl(用于编写3D游戏)或pyaudio(用于音频处理)等。
您可以使用PyCharm的内置命令行终端来安装这些模块。要打开命令行终端,请单击PyCharm底部的“终端”选项卡,然后运行上述pip命令即可。
相关问题
pycharm写猜拳小游戏
好的,下面是一个简单的猜拳小游戏的代码:
```python
import random # 导入random模块
print("欢迎来到猜拳游戏!")
while True:
print("请出拳:1.石头 2.剪刀 3.布")
user_choice = int(input()) # 获取用户输入
# 判断用户输入是否合法
if user_choice < 1 or user_choice > 3:
print("输入有误,请重新输入!")
continue
# 计算机随机出拳
computer_choice = random.randint(1, 3)
# 输出猜拳结果
if user_choice == computer_choice:
print("平局!")
elif (user_choice == 1 and computer_choice == 2) \
or (user_choice == 2 and computer_choice == 3) \
or (user_choice == 3 and computer_choice == 1):
print("恭喜你,你赢了!")
else:
print("很遗憾,你输了!")
# 询问用户是否继续游戏
print("是否继续游戏?(y/n)")
choice = input()
if choice == 'n':
break
print("游戏结束,谢谢参与!")
```
这个小游戏的思路很简单,首先输出欢迎语句,然后进入一个无限循环,每次循环中让用户出拳(通过输入数字1、2、3来表示出石头、剪刀、布),计算机随机出拳,比较两者的出拳结果,输出胜负信息。最后询问用户是否继续游戏,如果选择退出,则跳出循环,输出结束语句,游戏结束。
pycharm小游戏
PyCharm是一款流行的Python集成开发环境(IDE),它提供了丰富的功能和工具来方便开发者编写、调试和运行Python代码。使用PyCharm,你可以轻松地创建Python小游戏。
要创建一个简单的PyCharm小游戏,你可以按照以下步骤进行:
1. 在PyCharm中创建一个新的Python项目。
2. 在项目中创建一个Python文件,命名为你想要的游戏名称。
3. 导入所需的模块,如pygame。
4. 编写游戏逻辑代码,包括初始化游戏、游戏循环、事件处理、更新和绘制等。
5. 运行游戏代码,查看游戏效果。
在编写小游戏时,你可以利用pygame库来处理图形、声音和用户交互等方面。它为你提供了一系列方便的函数和工具,可以快速构建基于Python的游戏。
希望这些信息对你有帮助!如果你有任何具体的问题或需要更多的指导,请随时告诉我。
阅读全文
相关推荐
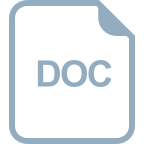
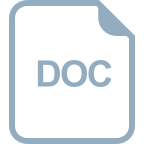
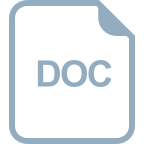
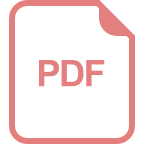












