我把一个含有多个字符串的C结构体转换为void*类型,然后把这个结构体给到go里的回调函数,我怎么把这个结构体再转换成go的结构体
时间: 2023-06-26 19:09:46 浏览: 74
在将C结构体转换为void指针时,你需要确保该指针包含足够的信息来重建原始结构体。如果你只是将指针转换为void指针而没有进行任何更改,那么你可以在Go中使用`unsafe.Pointer`将其转换回原始指针类型,然后将其强制转换为相应的Go结构体类型。
以下是一个示例代码,其中假设我们有一个名为`CStruct`的C结构体,其中包含一个字符串和一个整数,我们将其转换为void指针,然后将其传递给一个名为`Callback`的Go回调函数:
```c
#include <stdio.h>
// 定义包含字符串和整数的C结构体
typedef struct {
char str[10];
int num;
} CStruct;
// 将C结构体转换为void指针并传递给Go回调函数
void call_callback(void* data, void (*callback)(void*)) {
callback(data);
}
// Go回调函数,将void指针转换为Go结构体
void callback(void* data) {
CStruct* cstruct = (CStruct*)data;
printf("String: %s, Number: %d\n", cstruct->str, cstruct->num);
// 转换为Go结构体
// 注意:需要使用Cgo编译器
// #include "_cgo_export.h"
// GoStruct goStruct = ConvertToGoStruct(cstruct->str, cstruct->num);
}
int main() {
// 创建C结构体
CStruct cstruct = {"Hello", 123};
// 将C结构体转换为void指针并传递给Go回调函数
call_callback(&cstruct, callback);
return 0;
}
```
在Go中,你需要使用`//export`指令导出一个名为`ConvertToGoStruct`的C函数,该函数将接收C结构体字段的值并将其转换为Go结构体。然后,你可以在Go中包含生成的头文件`_cgo_export.h`,并调用`ConvertToGoStruct`函数来将C结构体转换为Go结构体。
以下是一个示例Go代码:
```go
package main
import "C"
import (
"unsafe"
)
// 定义与C结构体相同的Go结构体
type GoStruct struct {
Str string
Num int
}
// 导出的C函数,将C结构体转换为Go结构体
//export ConvertToGoStruct
func ConvertToGoStruct(str *C.char, num C.int) GoStruct {
goStr := C.GoString(str)
return GoStruct{goStr, int(num)}
}
func main() {
// 创建C结构体
cstruct := C.struct_CStruct{str: "Hello", num: 123}
// 将C结构体转换为void指针并传递给Go回调函数
C.call_callback(unsafe.Pointer(&cstruct), (*[0]byte)(unsafe.Pointer(C.callback)))
}
```
注意:在Go中调用C函数需要使用Cgo编译器。你需要在构建命令中包含`-buildmode=c-shared`标志,以便将Go代码编译为动态链接库,该库可以被C代码链接和使用。
相关推荐
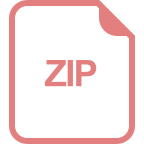
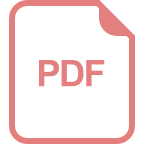
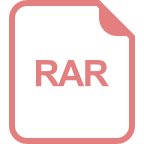
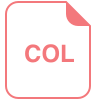
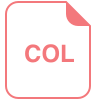
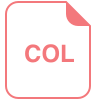
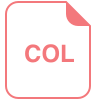
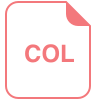









