pyqt5和yolov5结合
时间: 2023-06-29 11:13:12 浏览: 117
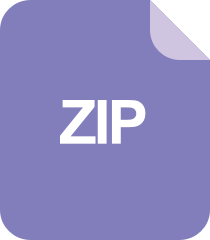
pyqt+yolov5+pcb缺陷检测,登入界面+支持图像视频检测整体为YoloV5的代码

PyQt5是一个用于创建GUI应用程序的Python库,而YoloV5是一个用于目标检测的深度学习模型。如果你想将它们结合起来,可以使用PyQt5创建一个GUI界面来显示YoloV5检测到的结果,并提供一些控制和交互功能。
以下是一个简单的示例代码,用于在GUI界面中显示YoloV5检测到的结果:
```python
import sys
import cv2
import numpy as np
from PyQt5.QtGui import QImage, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel, QWidget
from PyQt5.QtCore import Qt
# 导入yolov5模型和配置
sys.path.append('yolov5')
from models.experimental import attempt_load
from utils.general import check_img_size, non_max_suppression, scale_coords
from utils.datasets import letterbox
from utils.plots import plot_one_box
class YoloWidget(QWidget):
def __init__(self):
super().__init__()
self.label = QLabel(self)
self.label.setAlignment(Qt.AlignCenter)
self.setGeometry(100, 100, 800, 600)
self.setWindowTitle('YoloV5 Demo')
# 加载yolov5模型
self.model = attempt_load('yolov5s.pt', map_location='cpu')
def detect_objects(self, image_path):
# 加载图像并调整大小
img0 = cv2.imread(image_path)
img = letterbox(img0, new_shape=self.model.img_size)[0]
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
# 模型推理
img = torch.from_numpy(img).to(torch.float32)
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
pred = self.model(img)[0]
pred = non_max_suppression(pred, 0.4, 0.5)
# 绘制检测结果
for i, det in enumerate(pred):
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img0.shape).round()
for *xyxy, conf, cls in reversed(det):
c = int(cls)
label = f'{self.model.names[c]} {conf:.2f}'
plot_one_box(xyxy, img0, label=label, color=colors(c, True), line_thickness=3)
# 显示图像
height, width, channel = img0.shape
bytesPerLine = 3 * width
qimage = QImage(img0.data, width, height, bytesPerLine, QImage.Format_RGB888)
pixmap = QPixmap.fromImage(qimage)
self.label.setPixmap(pixmap)
self.label.resize(self.label.pixmap().size())
if __name__ == '__main__':
app = QApplication(sys.argv)
w = YoloWidget()
w.detect_objects('image.jpg')
w.show()
sys.exit(app.exec_())
```
在这个例子中,我们创建了一个名为`YoloWidget`的自定义QWidget,它显示指定图像路径的检测结果。我们使用YoloV5模型对图像进行推理,并使用OpenCV将检测结果绘制在原始图像上。然后,我们使用PyQt5将图像显示在GUI界面中。你可以根据自己的需求添加更多的控制和交互功能。
阅读全文
相关推荐
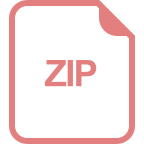
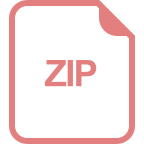
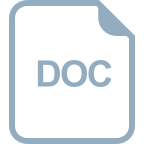
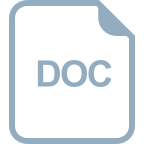
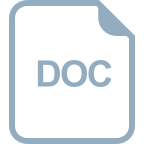
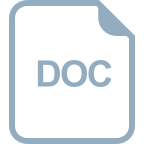
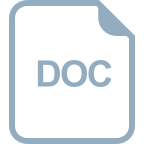
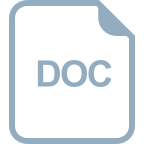
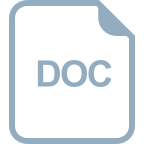
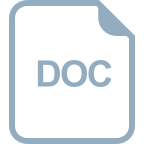
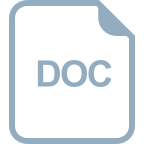



