"custom_relation.csv"
时间: 2023-07-07 19:39:02 浏览: 88
好的,文件路径已确认。下面是代码实现:
```python
import csv
# 创建一个名为relation的空字典
relation = {}
# 打开csv文件并读取数据
with open("custom_relation.csv", newline='', encoding='utf-8') as csvfile:
reader = csv.reader(csvfile)
next(reader) # 跳过首行
for row in reader:
# 将csv文件中的每一行数据添加到relation字典中
key = row[0]
value = row[1:]
relation[key] = value
```
上述代码会读取名为custom_relation.csv的文件,并将其中的数据存储在一个名为relation的字典中。请确保该csv文件与代码文件在同一目录下,并且csv文件中第一行为表头,不包含数据。
相关问题
小明想用pandas分析某公司的客户关系,数据文件存储在 custom_relation.csv文件中。 (1)请导入必要的包,并读取custom_relation.csv文件,数据赋值给relation对象。(5分) (2)显示relation对象前十行数据;查看relation的基本统计特性;查看relation对象的维度;打印全部的列名称。(10分) (3)‘Total day charge’表示每日消费额,请按每日消费额排序,并按照消费额从大到小输出。(5分) (4)使用loc方法输出0至10行,State,Total day minutes的数据。(5分) (5)请按照State进行分组求和,并将结果赋值给sc,按‘Total day charge’从大到小排序并输出State, Total day charge, Total eve charge。(10分) (6)使用柱状图打印输出sc。
好的,让我来一步一步回答你的问题:
(1) 导入必要的包,并读取 custom_relation.csv 文件,数据赋值给 relation 对象:
```python
import pandas as pd
# 读取数据文件
relation = pd.read_csv('custom_relation.csv')
```
(2) 显示 relation 对象前十行数据;查看 relation 的基本统计特性;查看 relation 对象的维度;打印全部的列名称:
```python
# 显示前十行数据
print(relation.head(10))
# 查看基本统计特性
print(relation.describe())
# 查看对象维度
print(relation.shape)
# 打印全部列名称
print(relation.columns)
```
(3) ‘Total day charge’ 表示每日消费额,请按每日消费额排序,并按照消费额从大到小输出:
```python
# 按每日消费额排序
relation.sort_values('Total day charge', ascending=False, inplace=True)
# 输出
print(relation[['Total day charge']])
```
(4) 使用 loc 方法输出 0 至 10 行,State,Total day minutes 的数据:
```python
# 使用 loc 方法输出 0 至 10 行,State,Total day minutes 的数据
print(relation.loc[0:10, ['State', 'Total day minutes']])
```
(5) 请按照 State 进行分组求和,并将结果赋值给 sc,按 ‘Total day charge’ 从大到小排序并输出 State, Total day charge, Total eve charge:
```python
# 按照 State 进行分组求和,并将结果赋值给 sc
sc = relation.groupby('State').sum()
# 按 Total day charge 从大到小排序并输出 State, Total day charge, Total eve charge
print(sc[['Total day charge', 'Total eve charge']].sort_values('Total day charge', ascending=False))
```
(6) 使用柱状图打印输出 sc:
```python
# 导入绘图包
import matplotlib.pyplot as plt
# 使用柱状图打印输出 sc
sc.plot(kind='bar', y='Total day charge')
plt.show()
```
好了,以上就是你提出的问题的完整解答啦!
h_list = [] t_list = [] r_list = [] self.train_kg_dict = collections.defaultdict(list) self.train_relation_dict = collections.defaultdict(list) for row in self.kg_train_data.iterrows(): h, r, t = row[1] h_list.append(h) t_list.append(t) r_list.append(r) self.train_kg_dict[h].append((t, r)) self.train_relation_dict[r].append((h, t)) self.h_list = torch.LongTensor(h_list) self.t_list = torch.LongTensor(t_list) self.r_list = torch.LongTensor(r_list)
这段代码看起来像是一个数据处理的部分,有些细节我可能看不出来,但是大致意思是将三元组(头实体,关系,尾实体)从kg_train_data中提取出来,并将它们分别存储在h_list、r_list、t_list三个列表中。同时,还将头实体h作为键,对应的值是一个包含(t, r)元组的列表train_kg_dict[h],表示以h为头实体的所有三元组。同理,将关系r作为键,对应的值是一个包含(h, t)元组的列表train_relation_dict[r],表示所有关系为r的三元组。最后,将h_list、t_list、r_list转化为PyTorch的张量分别存储在self.h_list、self.t_list、self.r_list中。
阅读全文
相关推荐
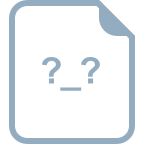
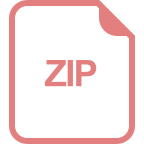










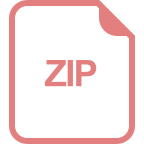

