利用opencv中dnn模型,给定一张图片,识别人脸并且画出可信度
时间: 2024-01-16 22:04:51 浏览: 35
好的,这是一个关于OpenCV中dnn模型的问题,我会为你解答。
首先,你需要选择一个人脸检测模型。OpenCV中的dnn模块支持多种深度学习框架的模型,比如Caffe、TensorFlow等,你可以选择其中任意一种。在这里,我将以使用Caffe框架的SSD人脸检测器为例进行讲解。
现在,我们假设你已经安装好了OpenCV和Caffe,并且已经下载好了训练好的SSD模型文件。接下来,我们需要编写一段代码来实现人脸检测并画出可信度。
```python
import cv2
# 加载模型
prototxt_path = "deploy.prototxt"
model_path = "res10_300x300_ssd_iter_140000.caffemodel"
net = cv2.dnn.readNetFromCaffe(prototxt_path, model_path)
# 加载图片
image = cv2.imread("image.jpg")
# 对图片进行预处理
blob = cv2.dnn.blobFromImage(cv2.resize(image, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
# 输入模型进行前向推理
net.setInput(blob)
detections = net.forward()
# 遍历检测结果并画出边框及可信度
for i in range(detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5: # 过滤掉置信度低的结果
box = detections[0, 0, i, 3:7] * np.array([image.shape[1], image.shape[0], image.shape[1], image.shape[0]])
(startX, startY, endX, endY) = box.astype("int")
cv2.rectangle(image, (startX, startY), (endX, endY), (0, 0, 255), 2)
text = "{:.2f}%".format(confidence * 100)
y = startY - 10 if startY - 10 > 10 else startY + 10
cv2.putText(image, text, (startX, y), cv2.FONT_HERSHEY_SIMPLEX, 0.45, (0, 0, 255), 2)
# 显示结果
cv2.imshow("Output", image)
cv2.waitKey(0)
```
在这段代码中,我们首先加载了SSD模型文件,然后读入了一张图片,接着对图片进行了预处理,将其转换为模型输入所需的格式。之后,我们将处理后的图片输入到模型中进行前向推理,得到了检测结果,遍历结果并画出边框及可信度。最后,我们显示了处理后的图片,等待用户按下任意键退出。
希望这个例子能够帮助你了解如何使用OpenCV中的dnn模块进行人脸检测并画出可信度。
相关推荐
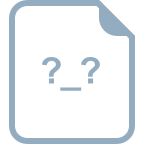
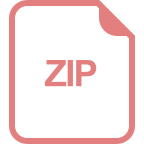

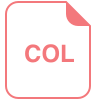
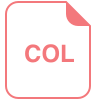
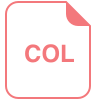
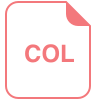









