建立一个链表每个结点包括的成员为:职工号、工资。用malloc打印出来。用一个creat 来建立链表,用list来输出数据。5个职工号为101,103,105,107,109。
时间: 2024-02-20 14:56:57 浏览: 120
好的,我可以帮你解决这个问题。以下是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct Employee {
int id; // 职工号
float salary; // 工资
struct Employee* next; // 指向下一个结点的指针
} Employee;
// 创建链表
Employee* creat() {
Employee* head = NULL; // 头结点指针
Employee* tail = NULL; // 尾结点指针
int ids[] = {101, 103, 105, 107, 109}; // 职工号数组
for (int i = 0; i < 5; i++) {
Employee* node = (Employee*)malloc(sizeof(Employee)); // 创建新结点
node->id = ids[i];
node->salary = 2000 * (i+1); // 工资为 2000, 4000, 6000, 8000, 10000
node->next = NULL;
if (head == NULL) { // 如果链表为空,头结点指针指向新结点
head = node;
} else { // 如果链表不为空,尾结点指针指向新结点
tail->next = node;
}
tail = node; // 尾结点指针指向新结点
}
return head;
}
// 输出链表
void list(Employee* head) {
Employee* p = head;
while (p != NULL) {
printf("职工号:%d,工资:%.2f\n", p->id, p->salary);
p = p->next;
}
}
// 主函数
int main() {
Employee* head = creat(); // 创建链表
list(head); // 输出链表
return 0;
}
```
这段代码先定义了一个名为 `Employee` 的结构体,用于表示链表中的每个结点。然后在 `creat()` 函数中创建了一个包含 5 个结点的链表,每个结点包括职工号和工资信息。最后在 `list()` 函数中输出了链表中所有结点的信息。在 `main()` 函数中调用 `creat()` 和 `list()` 函数,实现了整个程序的功能。
阅读全文
相关推荐
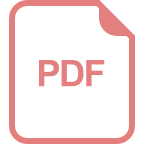
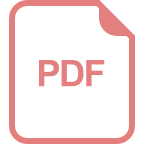
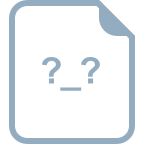













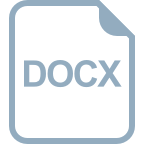
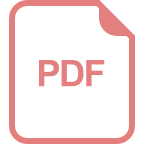
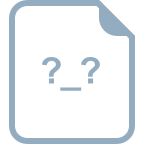